C Exercises: Display the first 10 catalan numbers
Write a program in C to display the first 10 Catalan numbers.
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
// Function to calculate the nth Catalan number recursively
unsigned long int cataLan(unsigned int n)
{
if (n <= 1) return 1; // Base case: Catalan number is 1 if n is 0 or 1
unsigned long int catno = 0; // Initialize the Catalan number
// Calculate the Catalan number using recursive formula
for (int i = 0; i < n; i++)
catno += cataLan(i) * cataLan(n - i - 1); // Sum of products of Catalan numbers
return catno; // Return the calculated Catalan number
}
int main()
{
printf("\n\n Find the first 10 Catalan numbers: \n");
printf(" --------------------------------------\n");
printf(" The first 10 Catalan numbers are: \n");
// Calculate and display the first 10 Catalan numbers
for (int i = 0; i < 10; i++)
printf("%lu ", cataLan(i)); // Print the ith Catalan number
printf("\n");
return 0;
}
Sample Output:
The first 10 catalan numbers are: 1 1 2 5 14 42 132 429 1430 4862
Visual Presentation:
Flowchart:
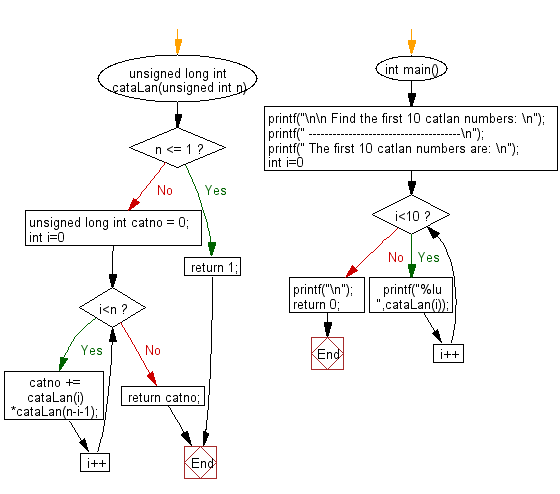
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to display the first 10 lucus numbers.
Next: Write a program in C to check a number is a Happy or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics