C Exercises: Check whether a given number is an ugly number
1. Ugly Number Variants
Write a program in C to check whether a given number is an ugly number or not.
Test Data
Input an integer number: 25
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
int main()
{
int n, x = 0; // Declaration of variables 'n' and 'x' to store the input number and flag respectively
printf("\n\n Check whether a given number is an ugly number:\n");
printf("----------------------------------------------------\n");
printf("Input an integer number: ");
scanf("%d", &n); // Read an integer from the user and store it in variable 'n'
if (n <= 0) {
printf("Input a correct number."); // If the entered number is less than or equal to zero, prompt the user to input a correct number
}
while (n != 1) { // Loop to check if the number is an ugly number
if (n % 5 == 0) {
n /= 5; // If the number is divisible by 5, divide it by 5
} else if (n % 3 == 0) {
n /= 3; // If the number is divisible by 3, divide it by 3
} else if (n % 2 == 0) {
n /= 2; // If the number is divisible by 2, divide it by 2
} else {
printf("It is not an ugly number.\n"); // If the number is not divisible by 2, 3, or 5, it's not an ugly number
x = 1; // Set flag 'x' to 1 to indicate it's not an ugly number
break; // Exit the loop
}
}
if (x == 0) { // Check if the flag 'x' is still 0 after the while loop
printf("It is an ugly number.\n"); // If 'x' is 0, then it's an ugly number
}
}
Sample Output:
Input an integer number: 25 It is an ugly number.
Visual Presentation:
Flowchart:
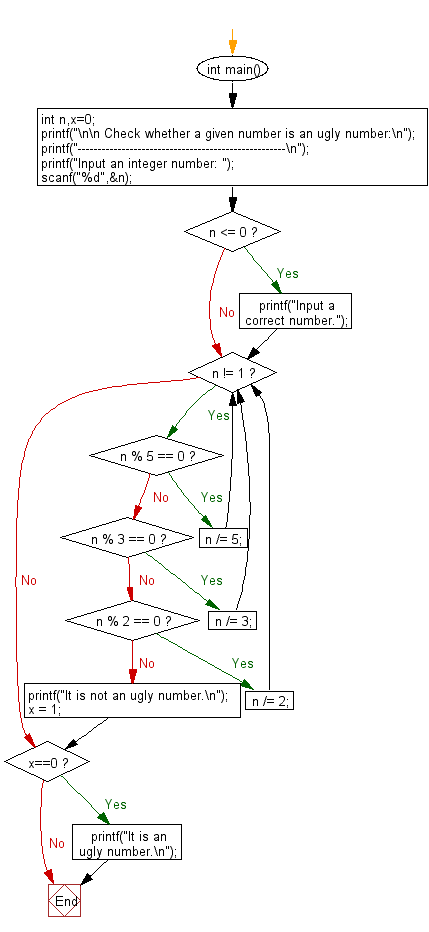
For more Practice: Solve these Related Problems:
- Write a C program to check if a number is ugly and then print its prime factors.
- Write a C program to generate the first n ugly numbers using dynamic programming.
- Write a C program to test if a number remains ugly after removing its trailing zeros.
- Write a C program to count the frequency of ugly numbers in a given range.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: C Programming Exercises on Numbers Home
Next: Write a program in C to check whether a given number is Abundant or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.