C Exercises: Calculate x raised to the power n
4. Power Function Variants
Write a C program to calculate x raised to the power n (xn).
Example:
Input:
x = 7.0
n = 2
x = 6.2
n = 3
Output:
Result:(x^n) : 49.000000
Result:(x^n) : 238.328000
Visual Presentation:
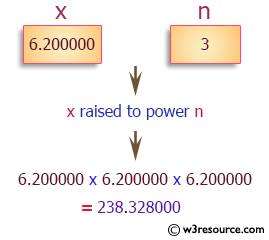
Sample Solution:
C Code:
#include <stdio.h>
#include <limits.h>
// Function to calculate power of x raised to the power n (x^n)
double powxn(double x, int n) {
double k;
// Base case: if n is 0, return 1
if (n == 0)
return 1;
// Recursive calculation of power using divide-and-conquer approach
k = powxn(x * x, n / 2); // Recursively compute x^(n/2)
// If n is odd, multiply k by x once more
if (n % 2)
k = k * x;
return k; // Return the computed value of x^n
}
int main(void)
{
double x = 7.0;
int n = 2;
// Test case 1
printf("\nx = %f, y = %d ", x, n);
printf("\nResult: (x^n) : %f ", powxn(x, n));
x = 6.2;
n = 3;
// Test case 2
printf("\n\nx = %f, y = %d ", x, n);
printf("\nResult: (x^n) : %f ", powxn(x, n));
return 0;
}
Sample Output:
x = 7.000000, y = 2 Result:(x^n) : 49.000000 x = 6.200000, y = 3 Result:(x^n) : 238.328000
Flowchart:
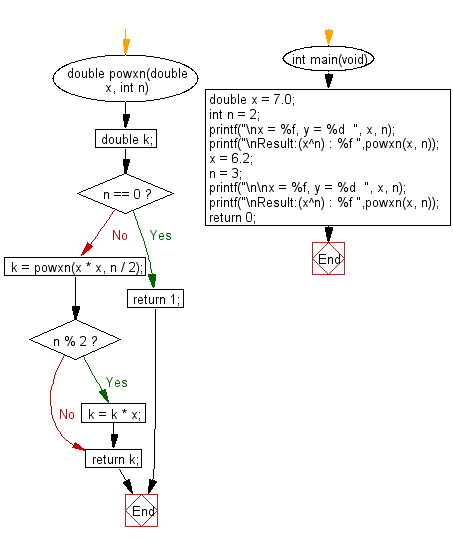
For more Practice: Solve these Related Problems:
- Write a C program to calculate x raised to n using recursion with exponentiation by squaring.
- Write a C program to compute x^n iteratively while handling negative exponents correctly.
- Write a C program to implement a power function without using the standard math library, using only loops and multiplication.
- Write a C program to compute x^n using recursion and tail-call optimization to reduce stack usage.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to check whether an integer is a palindrome or not.
Next: Write a C program to get the kth permutation sequence from two given integers n and k.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.