C Exercises: Divide two integers without using multiplication, division and mod operator
3. Division Without Operators Variants
Write a C program to divide two integers (dividend and divisor) without using the multiplication, division and mod operator.
Example:
Input:
dividend_num = 7
divisor_num = 2
dividend_num = -17
divisor_num = 5
dividend_num = 35
divisor_num = 7
Output:
Result: 3
Result: -3
Result: 5
Visual Presentation:
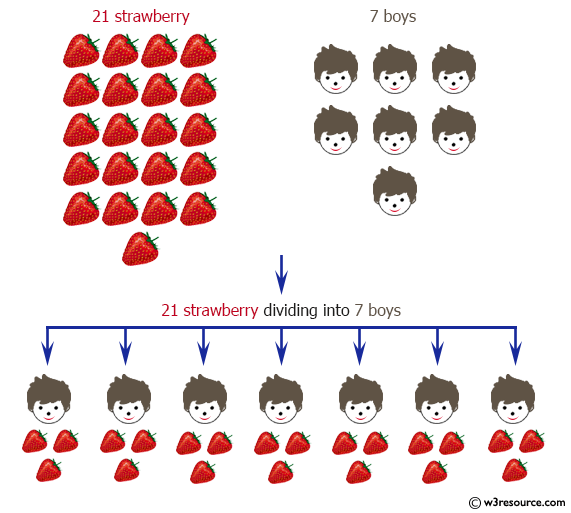
Sample Solution:
C Code:
#include <stdio.h>
#include <limits.h>
// Function to perform integer division without using the '/' operator
int divide_result(int dividend_num, int divisor_num) {
int sign = 1; // Variable to hold the sign of the result
long int output = 0; // Variable to store the result of division
// Handling sign of the numbers and converting to negative if necessary
if (dividend_num < 0) {
sign *= -1;
} else {
dividend_num *= -1;
}
if (divisor_num < 0) {
sign *= -1;
} else {
divisor_num *= -1;
}
// Performing division using subtraction
while (dividend_num <= divisor_num) {
long int temp = 0;
long int div = divisor_num;
// Repeatedly subtracting divisor from dividend until dividend is smaller
while (dividend_num <= div) {
temp += (temp + 1);
dividend_num -= div;
div += div;
}
// Checking for overflow and returning INT_MIN or INT_MAX accordingly
if (output >= INT_MAX) {
if (sign == -1) {
return INT_MIN;
} else {
return INT_MAX;
}
}
output += temp; // Adding the calculated value to the result
}
return output * sign; // Return the final result with proper sign
}
int main(void)
{
// Test cases for the divide_result function
int dividend_num = 7;
int divisor_num = 2;
printf("\nDividend %d, Divisor %d ", dividend_num, divisor_num);
printf("\nResult: %d ", divide_result(dividend_num, divisor_num));
dividend_num = -17;
divisor_num = 5;
printf("\n\nDividend %d, Divisor %d ", dividend_num, divisor_num);
printf("\nResult: %d ", divide_result(dividend_num, divisor_num));
dividend_num = 35;
divisor_num = 7;
printf("\n\nDividend %d, Divisor %d ", dividend_num, divisor_num);
printf("\nResult: %d ", divide_result(dividend_num, divisor_num));
return 0;
}
Sample Output:
Dividend 7, Divisior 2 Result: 3 Dividend -17, Divisior 5 Result: -3 Dividend 35, Divisior 7 Result: 5
Flowchart:
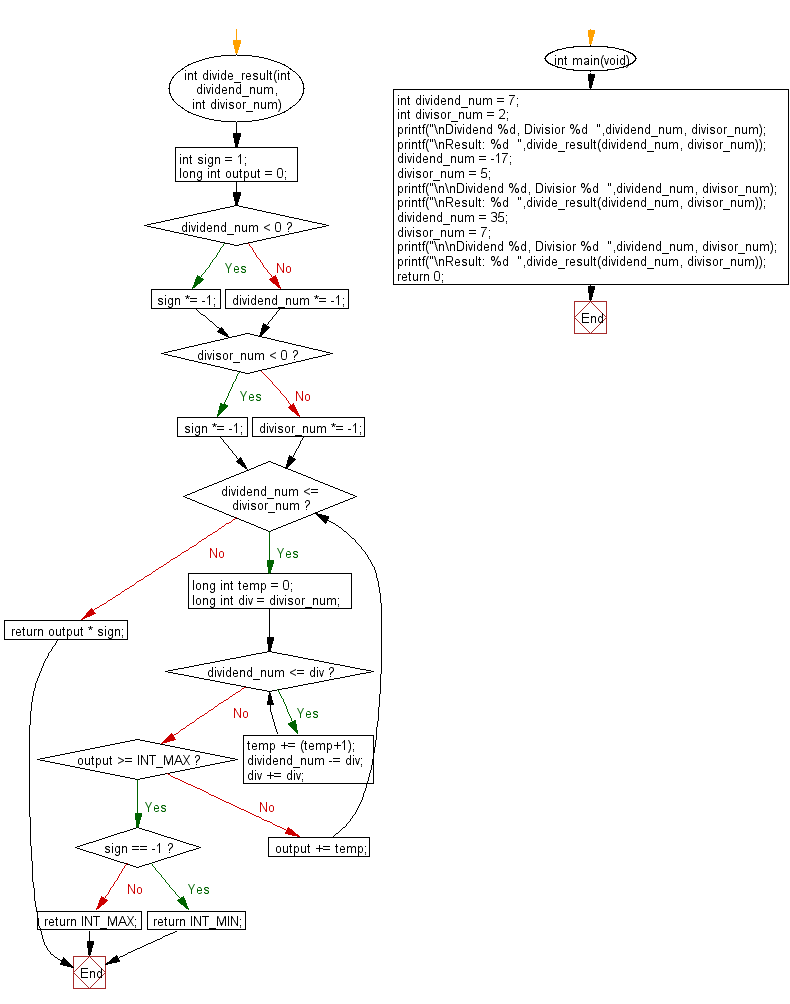
For more Practice: Solve these Related Problems:
- Write a C program to divide two integers using subtraction in a recursive manner.
- Write a C program to perform division without using multiplication, division, or modulus operators, but using addition and subtraction only.
- Write a C program to implement division using bit shifting and subtraction without direct multiplication or division.
- Write a C program to compute the quotient of two integers by simulating long division with loops and conditional checks.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to check whether an integer is a palindrome or not.
Next: Write a C program to calculate x raised to the power n (xn).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics