C Exercises: Calculate and print average of the stream of given numbers
21. Running Average Calculation Variants
Write a C program to calculate and print the average (or mean) of a stream of given numbers.
Example 1:
Input:
arr[] = {10, 20, 30, 40, 50, 60, 70, 80, 90, 100}
Output:
Average of 1 numbers is 10.000000
Average of 2 numbers is 15.000000
Average of 3 numbers is 20.000000
Average of 4 numbers is 25.000000
Average of 5 numbers is 30.000000
Average of 6 numbers is 35.000000
Average of 7 numbers is 40.000000
Average of 8 numbers is 45.000000
Average of 9 numbers is 50.000000
Average of 10 numbers is 55.000000
Visual Presentation:
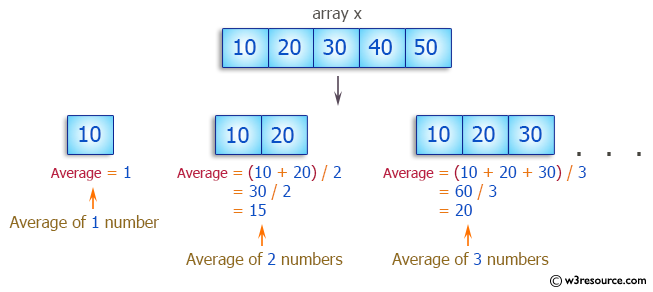
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function to compute streaming average of an array
void streamAvg(float arr[], int arr_size) {
float avg = 0; // Variable to store the average
int i = 0;
// Loop through the array to compute streaming averages
while (i < arr_size) {
avg = (avg * i + arr[i]) / (i + 1); // Update the average value
printf("\nAverage of %d numbers is %f \n", i + 1, avg); // Print the streaming average
i++;
}
return; // End of function
}
// Main function
int main(void) {
float arr[] = {10, 20, 30, 40, 50, 60, 70, 80, 90, 100}; // Array of floating point numbers
int arr_size = sizeof(arr) / sizeof(arr[0]); // Calculate the size of the array
printf("\nElements in array are: ");
int i = 0;
// Print the elements in the array
while (i < 10) {
printf("%f ", arr[i]);
i++;
}
streamAvg(arr, arr_size); // Call the function to calculate streaming averages
return 0; // End of the main function
}
Sample Output:
Elements in array are: 10.000000 20.000000 30.000000 40.000000 50.000000 60.000000 70.000000 80.000000 90.000000 100.000000 Average of 1 numbers is 10.000000 Average of 2 numbers is 15.000000 Average of 3 numbers is 20.000000 Average of 4 numbers is 25.000000 Average of 5 numbers is 30.000000 Average of 6 numbers is 35.000000 Average of 7 numbers is 40.000000 Average of 8 numbers is 45.000000 Average of 9 numbers is 50.000000 Average of 10 numbers is 55.000000
Flowchart:
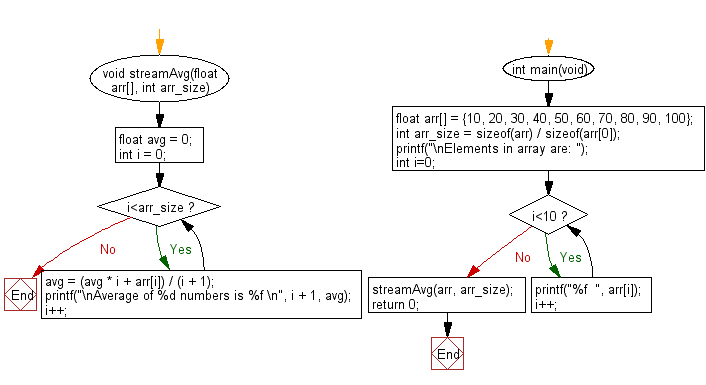
For more Practice: Solve these Related Problems:
- Write a C program to compute a running average of numbers input as a stream, updating after each new number.
- Write a C program to calculate cumulative averages and display the result after each addition using incremental formulas.
- Write a C program to read numbers from an array and print the average after processing each element sequentially.
- Write a C program to maintain a dynamic average with each new input without storing all previous numbers.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to multiply two integers without using multiplication, division, bitwise operators, and loops.
Next: Write a C program to count the numbers without digit 7, from 1 to a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.