C Exercises: Find the square root of a number using Babylonian method
19. Babylonian Square Root Variants
Write a C program to find the square root of a number using the Babylonian method.
Example 1:
Input: n = 50
Output: 7.071068
Example 2:
Input: n = 17
Output: 4.123106
Sample Solution:
C Code:
#include <stdio.h>
// Function to calculate the square root of a number using the Babylonian method
float square_Root(float n) {
float a = n; // Initial value for 'a' is the number itself
float b = 1; // Initial value for 'b' is 1
double e = 0.000001; // Threshold for the difference between 'a' and 'b'
// Loop to approximate the square root using the Babylonian method
while (a - b > e) {
a = (a + b) / 2; // Update 'a' by averaging 'a' and 'b'
b = n / a; // Calculate 'b' as the number divided by the updated 'a'
}
return a; // Return the calculated square root
}
// Main function to test the square_Root function with different values of 'n'
int main(void) {
int n = 50;
printf("Square root of %d is %f", n, square_Root(n)); // Print the square root of 'n'
n = 17;
printf("\nSquare root of %d is %f", n, square_Root(n)); // Print the square root of 'n'
return 0;
}
Sample Output:
Square root of 50 is 7.071068 Square root of 17 is 4.123106
Flowchart:
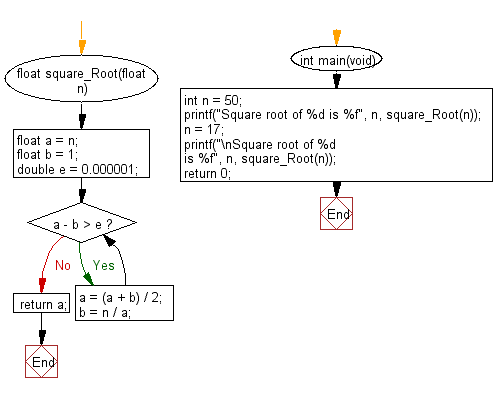
For more Practice: Solve these Related Problems:
- Write a C program to compute the square root of a number using the Babylonian method with a specified precision.
- Write a C program to implement the Babylonian method iteratively and count the number of iterations needed.
- Write a C program to compare the Babylonian square root approximation with the standard library function.
- Write a C program to calculate square roots using the Babylonian method and handle edge cases like zero and negative inputs.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C programming to find the total number of full staircase rows that can be formed from given number of dices.
Next: Write a C program to multiply two integers without using multiplication, division, bitwise operators, and loops.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.