C Exercises: Calculate the number of 1's in their binary representation and return them as an array
C Programming Mathematics: Exercise-14 with Solution
For a non negative integer in the range 0 ≤ i ≤ n write a C program to calculate the number of 1's in their binary representation and return them as an array.
Example:
Input:
Number: 7
Number of 1's in the binary representation:
0: 0
1: 1
2: 1
3: 2
4: 1
5: 2
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function to count the number of set bits (1s) in the binary representation of numbers from 0 to 'num'
int* count_Bits(int num, int* returnSize) {
int *p, i;
// Allocate memory for the array to store the count of set bits
p = malloc((num + 1) * sizeof(int));
*returnSize = num + 1; // Set the return size to 'num + 1'
p[0] = 0; // The count of set bits in 0 is 0
for (i = 1; i <= num; i++) {
// Use bitwise manipulation to count the set bits for each number
// Count the number of set bits by using the formula: p[i] = p[i & (i - 1)] + 1
p[i] = p[i & (i - 1)] + 1;
}
return p; // Return the array containing the count of set bits for numbers from 0 to 'num'
}
// Main function to test the count_Bits function and display the count of set bits for numbers up to 'num'
int main(void) {
int *p;
int returnSize;
int i = 7;
printf("Number: %d", i);
printf("\nNumber of 1's in the binary representation:\n");
// Calculate and display the count of set bits for numbers up to 5
p = count_Bits(5, &returnSize);
for (i = 0; i < returnSize; i++) {
printf("%X:\t%d\n", i, p[i]);
}
free(p); // Free the dynamically allocated memory
return 0;
}
Sample Output:
Number: 7 Number of 1's in the binary representation: 0: 0 1: 1 2: 1 3: 2 4: 1 5: 2
Flowchart:
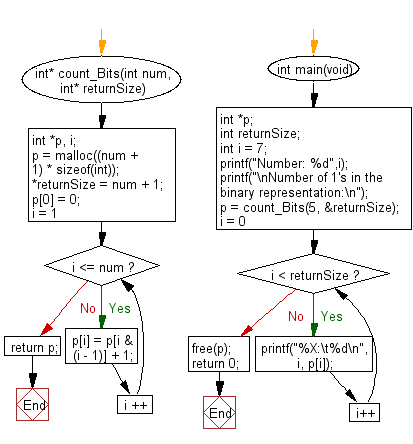
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C programming to check if a given integer is a power of three.
Next: Write a C programming to get the maximum product from a given integer after breaking the integer into the sum of at least two positive integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics