C Exercises: Delete a node from the middle of Singly Linked List
8. Middle Deletion Challenges
Write a program in C to delete a node from the middle of a Singly Linked List.
Visual Presentation:
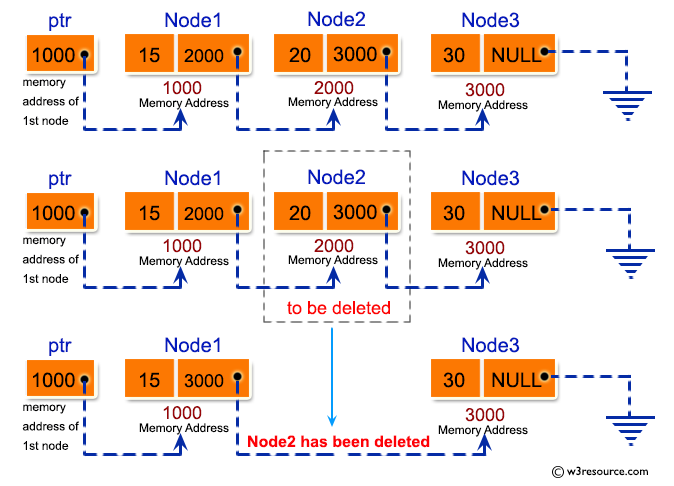
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
struct node
{
int num; // Data of the node
struct node *nextptr; // Address of the node
}*stnode; // Pointer to the starting node
// Function prototypes
void createNodeList(int n); // Function to create the linked list
void MiddleNodeDeletion(int pos); // Function to delete a node from the middle
void displayList(); // Function to display the list
// Main function
int main()
{
int n, num, pos;
printf("\n\n Linked List : Delete a node from the middle of Singly Linked List. :\n");
printf("-------------------------------------------------------------------------\n");
printf(" Input the number of nodes : ");
scanf("%d", &n);
createNodeList(n);
printf("\n Data entered in the list are : \n");
displayList();
printf("\n Input the position of node to delete : ");
scanf("%d", &pos);
if (pos <= 1 || pos >= n)
{
printf("\n Deletion can not be possible from that position.\n ");
}
if (pos > 1 && pos < n)
{
printf("\n Deletion completed successfully.\n ");
MiddleNodeDeletion(pos);
}
printf("\n The new list are : \n");
displayList();
return 0;
}
// Function to create a linked list with n nodes
void createNodeList(int n)
{
struct node *fnNode, *tmp;
int num, i;
stnode = (struct node *)malloc(sizeof(struct node));
if (stnode == NULL) // Check whether stnode is NULL for memory allocation
{
printf(" Memory can not be allocated.");
}
else
{
printf(" Input data for node 1 : ");
scanf("%d", &num);
stnode->num = num; // Assign data to the first node
stnode->nextptr = NULL; // Links the address field to NULL
tmp = stnode;
// Create n nodes and add to the linked list
for (i = 2; i <= n; i++)
{
fnNode = (struct node *)malloc(sizeof(struct node));
if (fnNode == NULL) // Check whether fnNode is NULL for memory allocation
{
printf(" Memory can not be allocated.");
break;
}
else
{
printf(" Input data for node %d : ", i);
scanf(" %d", &num);
fnNode->num = num; // Assign data to the current node
fnNode->nextptr = NULL; // Links the address field to NULL
tmp->nextptr = fnNode; // Links previous node i.e. tmp to the fnNode
tmp = tmp->nextptr;
}
}
}
}
// Function to delete a node from the middle of the list at a specific position
void MiddleNodeDeletion(int pos)
{
int i;
struct node *toDelMid, *preNode;
if (stnode == NULL)
{
printf(" There are no nodes in the List.");
}
else
{
toDelMid = stnode;
preNode = stnode;
// Traverse to the specified position in the list
for (i = 2; i <= pos; i++)
{
preNode = toDelMid;
toDelMid = toDelMid->nextptr;
if (toDelMid == NULL)
break;
}
if (toDelMid != NULL)
{
if (toDelMid == stnode) // If the node to delete is the first node
stnode = stnode->nextptr;
preNode->nextptr = toDelMid->nextptr; // Adjust the pointers
toDelMid->nextptr = NULL;
free(toDelMid); // Free memory of the deleted node
}
else
{
printf(" Deletion can not be possible from that position.");
}
}
}
// Function to display the linked list
void displayList()
{
struct node *tmp;
if (stnode == NULL)
{
printf(" No data found in the list.");
}
else
{
tmp = stnode;
while (tmp != NULL)
{
printf(" Data = %d\n", tmp->num); // Print the data of the current node
tmp = tmp->nextptr; // Move to the next node
}
}
}
Sample Output:
Linked List : delete a node from the middle of Singly Linked List. : ------------------------------------------------------------------------- Input the number of nodes : 3 Input data for node 1 : 2 Input data for node 2 : 5 Input data for node 3 : 8 Data entered in the list are : Data = 2 Data = 5 Data = 8 Input the position of node to delete : 2 Deletion completed successfully. The new list are : Data = 2 Data = 8
Flowchart:
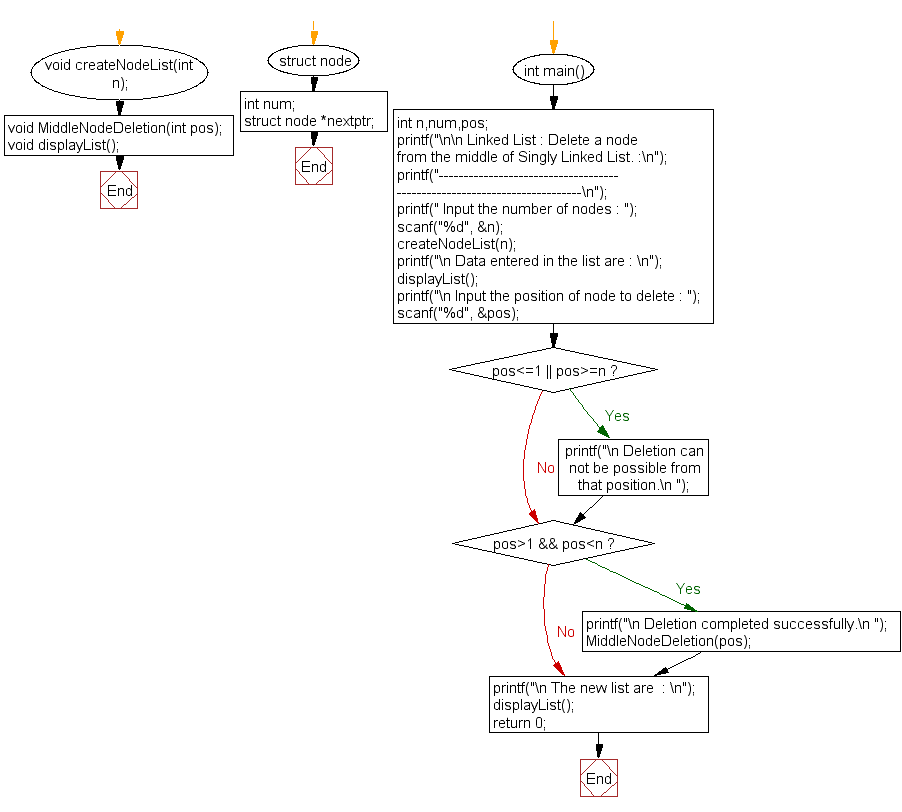
createNodeList() :
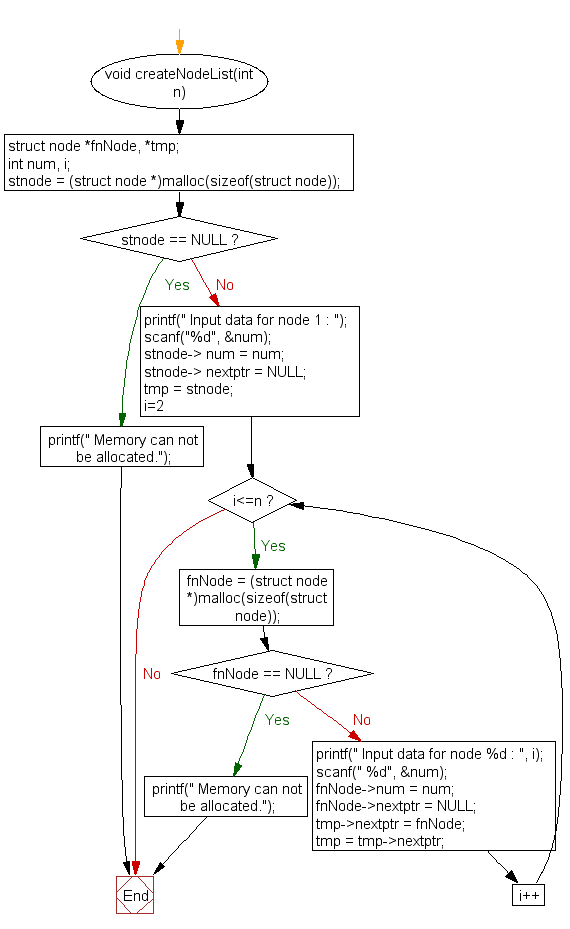
MiddleNodeDeletion() :
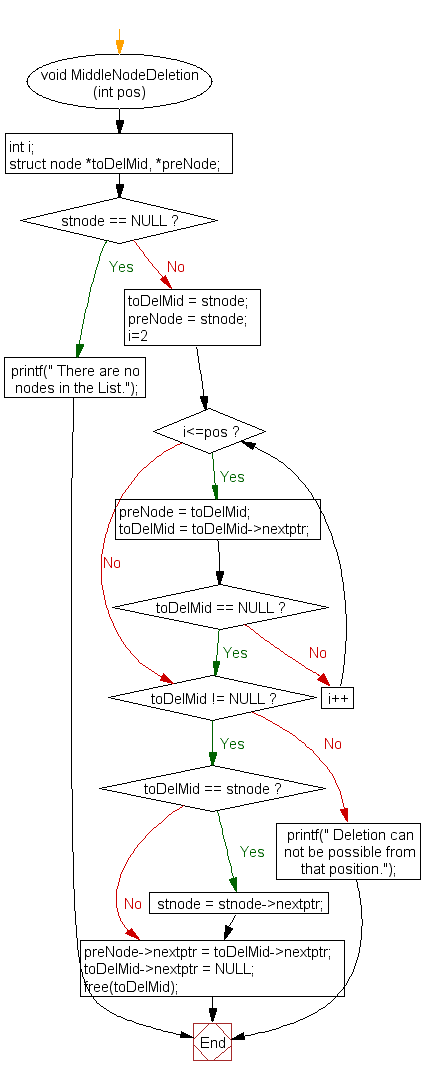
displayList() :
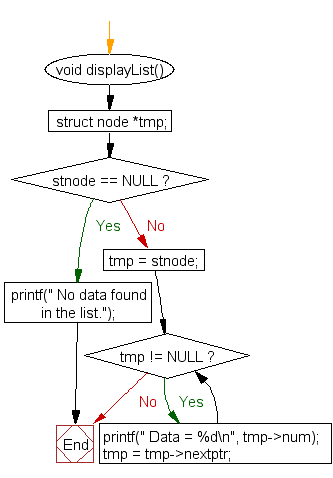
For more Practice: Solve these Related Problems:
- Write a C program to delete a node from a specific middle position provided by the user in a singly linked list.
- Write a C program to automatically delete the middle node of a linked list determined by its length.
- Write a C program to delete a middle node only if its value meets a given condition, such as being a prime number.
- Write a C program to delete two consecutive nodes from the middle of a linked list and then display the updated list.
C Programming Code Editor:
Previous: Write a program in C to delete first node of Singly Linked List.
Next: Write a program in C to delete the last node of Singly Linked List.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.