C Exercises: Reverse alternate k nodes of a singly linked list
41. Alternate K-Node Reversal Challenges
Write a C program to reverse alternate k nodes of a given singly linked list.
Sample Solution:
C Code:
Sample Output:
Original List: 1 2 3 4 5 6 7 8 Reverse alternate k (k=2) nodes of the said singly linked list: 2 1 3 4 6 5 7 8 Reverse alternate k (k=3) nodes of the said singly linked list: 3 1 2 4 6 5 8 7 Reverse alternate k (k=4) nodes of the said singly linked list: 4 2 1 3 6 5 8 7
Flowchart :
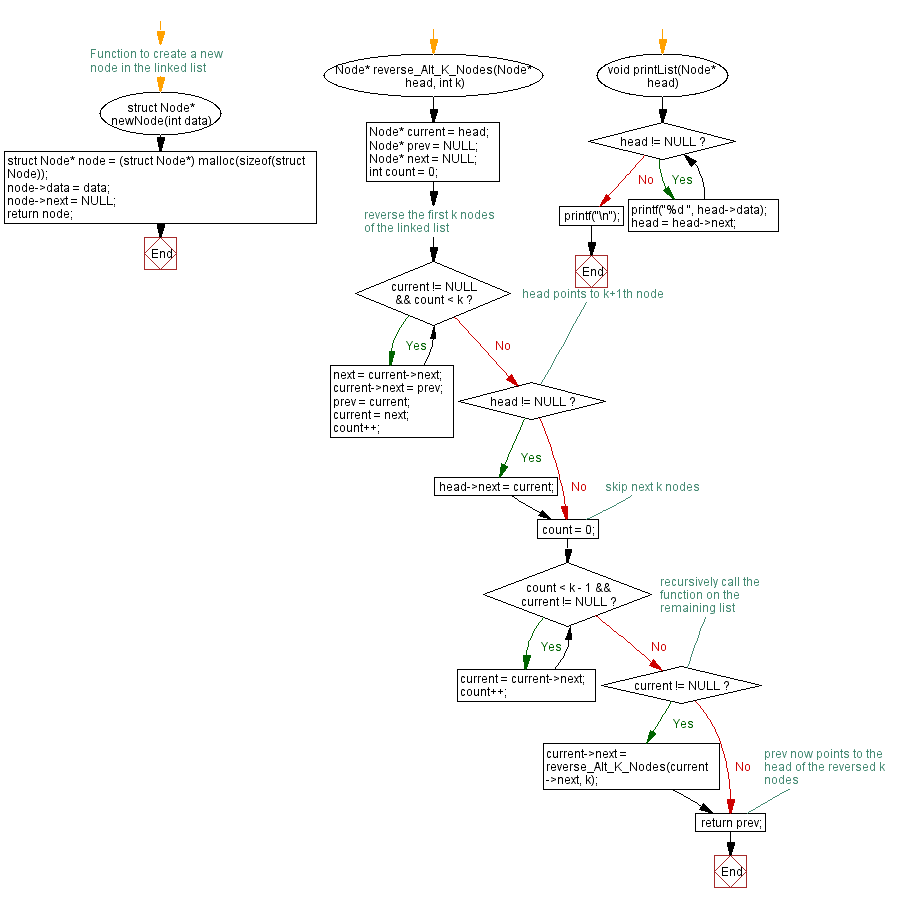
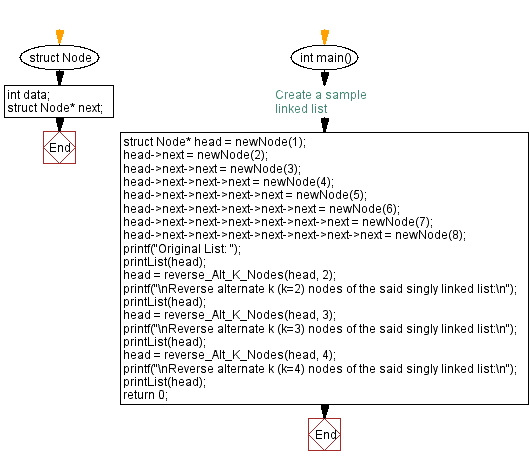
For more Practice: Solve these Related Problems:
- Write a C program to reverse alternate groups of k nodes in a linked list and then merge them with the unreversed groups in reverse order.
- Write a C program to reverse alternate k nodes recursively and then perform a pairwise swap on the reversed segments.
- Write a C program to reverse alternate groups of k nodes only if the sum of the nodes in the group is even.
- Write a C program to reverse alternate k nodes in a linked list and then rotate the entire list by k positions.
C Programming Code Editor:
Previous: Swap every two adjacent nodes of a singly linked list.
Next: Find the point at which two singly linked lists intersect.
What is the difficulty level of this exercise?