C Exercises: Insert a new node at the middle of the Linked List
C Singly Linked List : Exercise-6 with Solution
Write a program in C to insert a node in the middle of a Singly Linked List.
Visual Presentation:
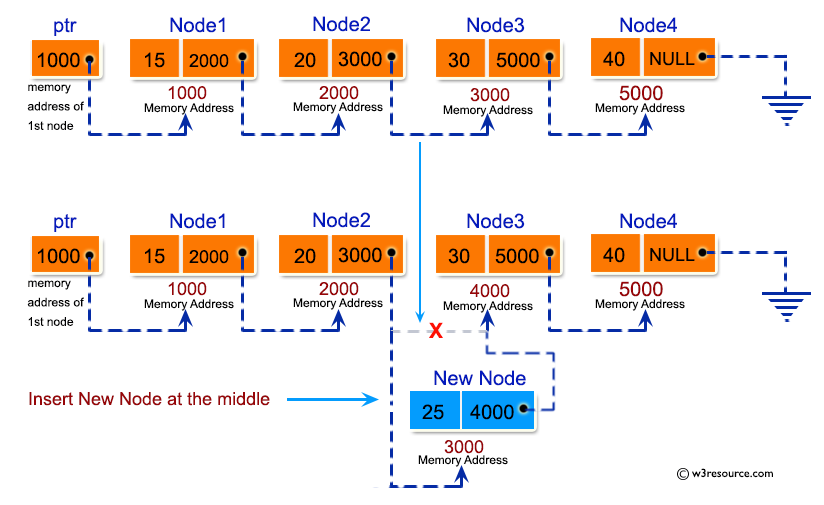
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Structure to define a node in a linked list
struct node
{
int num; // Data of the node
struct node *nextptr; // Address of the next node
}*stnode; // Pointer to the starting node
// Function prototypes
void createNodeList(int n); // Function to create the linked list
void insertNodeAtMiddle(int num, int pos); // Function to insert a node at a given position
void displayList(); // Function to display the linked list
// Main function
int main()
{
int n, num, pos;
// Displaying the purpose of the program
printf("\n\n Linked List : Insert a new node at the middle of the Linked List :\n");
printf("-----------------------------------------------------------------------\n");
// Inputting the number of nodes for the linked list
printf(" Input the number of nodes (3 or more) : ");
scanf("%d", &n);
// Creating the linked list with n nodes
createNodeList(n);
printf("\n Data entered in the list are : \n");
displayList();
// Inputting data and position to insert in the middle of the list
printf("\n Input data to insert in the middle of the list : ");
scanf("%d", &num);
printf(" Input the position to insert new node : ");
scanf("%d", &pos);
// Checking if insertion is possible at the given position
if(pos <= 1 || pos >= n)
{
printf("\n Insertion cannot be possible in that position.\n");
}
else if(pos > 1 && pos < n)
{
insertNodeAtMiddle(num, pos);
printf("\n Insertion completed successfully.\n");
}
// Displaying the updated list
printf("\n The new list is : \n");
displayList();
return 0;
}
// Function to create a linked list with n nodes
void createNodeList(int n)
{
struct node *fnNode, *tmp;
int num, i;
stnode = (struct node *)malloc(sizeof(struct node));
if(stnode == NULL) // Check whether stnode is NULL for memory allocation
{
printf(" Memory cannot be allocated.");
}
else
{
// Reading data for the first node
printf(" Input data for node 1 : ");
scanf("%d", &num);
stnode->num = num;
stnode->nextptr = NULL; // Links the address field to NULL
tmp = stnode;
// Creates n nodes and adds them to the linked list
for(i = 2; i <= n; i++)
{
fnNode = (struct node *)malloc(sizeof(struct node));
if(fnNode == NULL) // Check whether fnNode is NULL for memory allocation
{
printf(" Memory cannot be allocated.");
break;
}
else
{
printf(" Input data for node %d : ", i);
scanf(" %d", &num);
fnNode->num = num; // Links the num field of fnNode with num
fnNode->nextptr = NULL; // Links the address field of fnNode with NULL
tmp->nextptr = fnNode; // Links previous node i.e. tmp to the fnNode
tmp = tmp->nextptr;
}
}
}
}
// Function to insert a node at a specified position in the list
void insertNodeAtMiddle(int num, int pos)
{
int i;
struct node *fnNode, *tmp;
fnNode = (struct node*)malloc(sizeof(struct node));
if(fnNode == NULL) // Check whether fnNode is NULL for memory allocation
{
printf(" Memory cannot be allocated.");
}
else
{
fnNode->num = num; // Links the data part
fnNode->nextptr = NULL;
tmp = stnode;
for(i = 2; i <= pos - 1; i++)
{
tmp = tmp->nextptr;
if(tmp == NULL)
break;
}
if(tmp != NULL)
{
fnNode->nextptr = tmp->nextptr; // Links the address part of new node
tmp->nextptr = fnNode;
}
else
{
printf(" Insertion is not possible at the given position.\n");
}
}
}
// Function to display the linked list
void displayList()
{
struct node *tmp;
if(stnode == NULL)
{
printf(" No data found in the empty list.");
}
else
{
tmp = stnode;
while(tmp != NULL)
{
printf(" Data = %d\n", tmp->num); // Prints the data of current node
tmp = tmp->nextptr; // Advances the position of current node
}
}
}
Sample Output:
Linked List : Insert a new node at the middle of the Linked List : ----------------------------------------------------------------------- Input the number of nodes (3 or more) : 4 Input data for node 1 : 1 Input data for node 2 : 2 Input data for node 3 : 3 Input data for node 4 : 4 Data entered in the list are : Data = 1 Data = 2 Data = 3 Data = 4 Input data to insert in the middle of the list : 5 Input the position to insert new node : 3 Insertion completed successfully. The new list are : Data = 1 Data = 2 Data = 5 Data = 3 Data = 4
Flowchart:
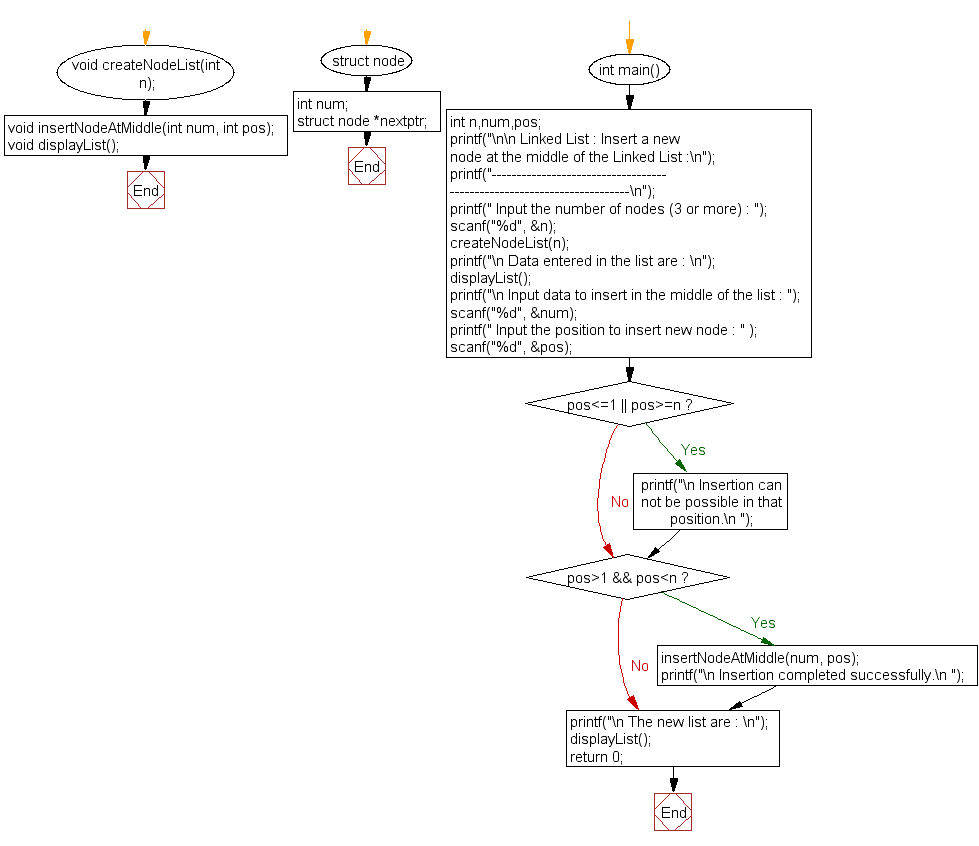
createNodeList():
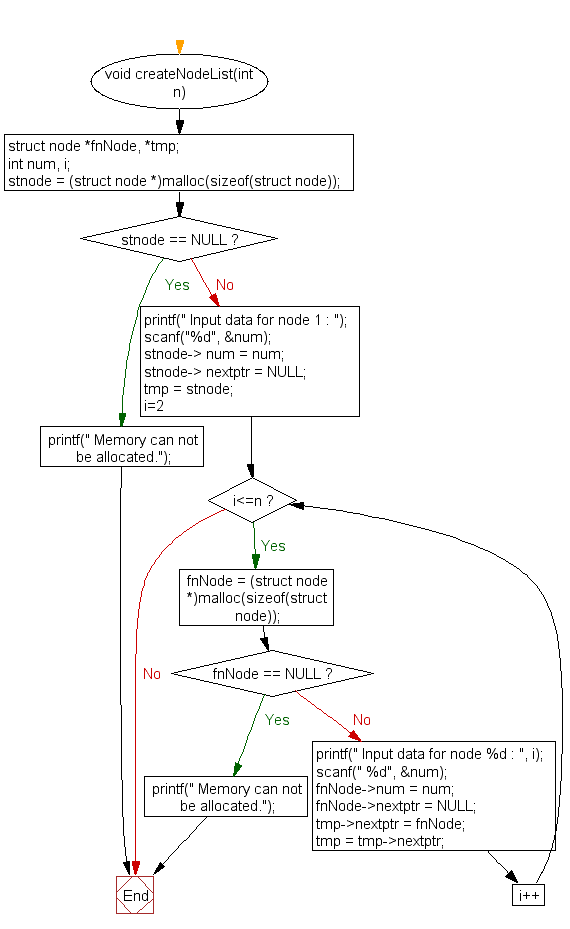
insertNodeAtMiddle() :
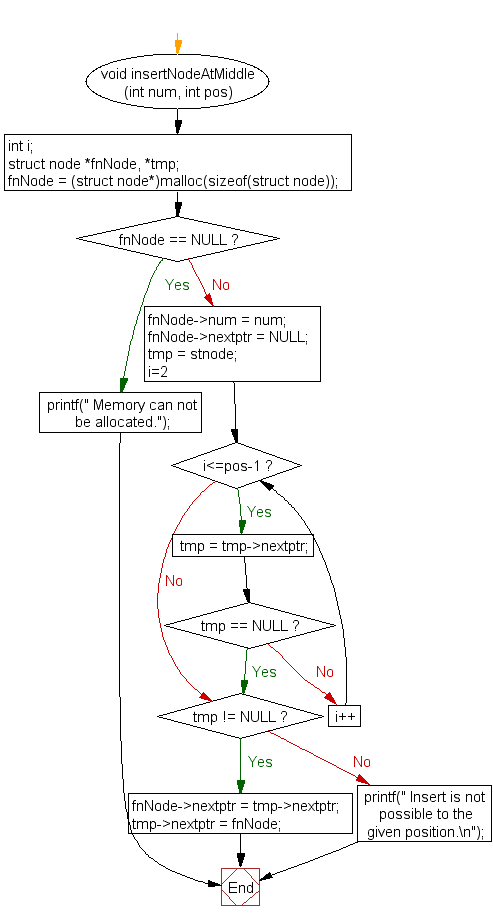
displayList() :
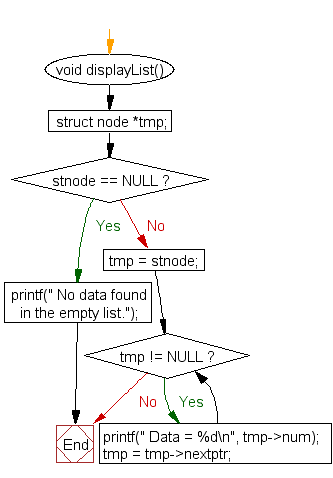
C Programming Code Editor:
Previous: Write a program in C to insert aNext: new node at the end of a Singly Linked List.
Write a program in C to delete first node of Singly Linked List.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/linked_list/c-linked_list-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics