C Exercises: Remove duplicates from a unsorted singly linked list
16. Duplicate Removal Challenges
Write a C program to remove duplicates from a single unsorted linked list.
Sample Solution:
C Code:
#include<stdio.h>
#include <stdlib.h>
// Define a structure for a Node in a singly linked list
struct Node {
int data;
struct Node* next;
};
// Function to create a new node with given data
struct Node* newNode(int data) {
// Allocate memory for a new node
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
// Set the data for the new node
node->data = data;
// Set the next pointer of the new node to NULL
node->next = NULL;
return node;
}
// Function to remove duplicates from a sorted linked list
void remove_Duplicates(struct Node* head) {
// Declare pointers to traverse the list
struct Node *current = head, *next_next;
// Traverse the list until the end is reached
while (current->next != NULL) {
// Check if the current node's value is equal to the next node's value
if (current->data == current->next->data) {
// Update pointers to remove the duplicate node
next_next = current->next->next;
free(current->next); // Free memory of the duplicate node
current->next = next_next; // Update the link to bypass the duplicate node
} else {
current = current->next; // Move to the next node
}
}
}
// Function to display the elements of a linked list
void displayList(struct Node* head) {
// Traverse the list and print each element
while (head) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
// Main function where the execution starts
int main() {
// Create the first linked list
struct Node* head1 = newNode(1);
head1->next = newNode(2);
head1->next->next = newNode(3);
head1->next->next->next = newNode(3);
head1->next->next->next->next = newNode(4);
printf("Original Singly List:\n");
displayList(head1); // Display the original list
printf("After removing duplicate elements from the said singly list:\n");
remove_Duplicates(head1); // Remove duplicates
displayList(head1); // Display the modified list without duplicates
// Create the second linked list
struct Node* head2 = newNode(1);
head2->next = newNode(2);
head2->next->next = newNode(3);
head2->next->next->next = newNode(3);
head2->next->next->next->next = newNode(4);
head2->next->next->next->next->next = newNode(4);
printf("\nOriginal Singly List:\n");
displayList(head2); // Display the original list
printf("After removing duplicate elements from the said singly list:\n");
remove_Duplicates(head2); // Remove duplicates
displayList(head2); // Display the modified list without duplicates
return 0; // Indicates successful completion of the program
}
Sample Output:
Original Singly List: 1 2 3 3 4 After removing duplicate elements from the said singly list: 1 2 3 4 Original Singly List: 1 2 3 3 4 4 After removing duplicate elements from the said singly list: 1 2 3 4
Flowchart :
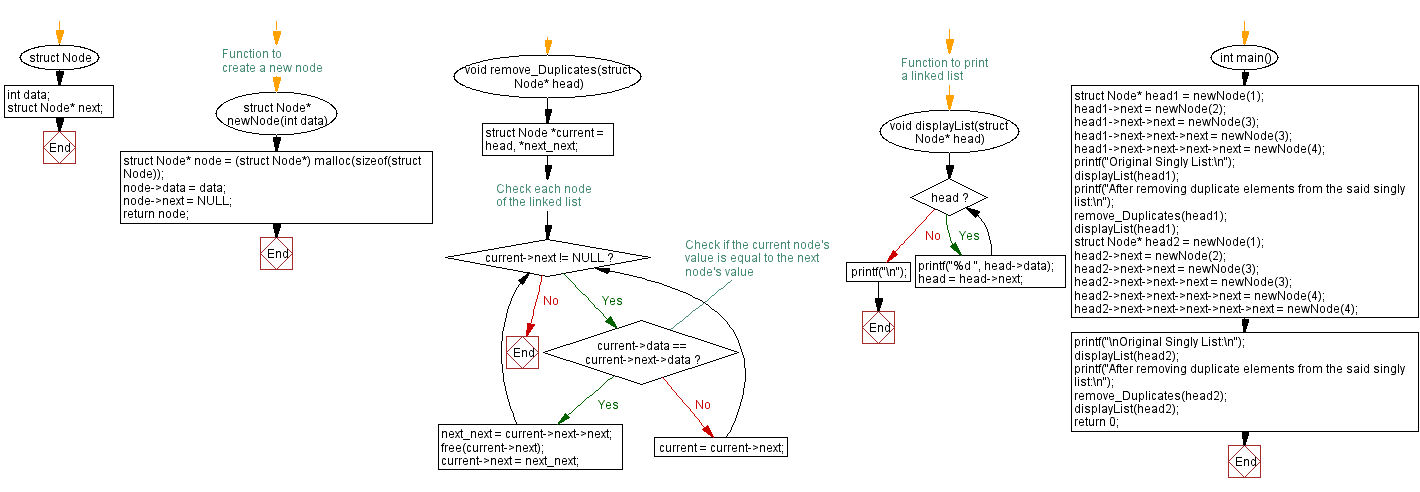
For more Practice: Solve these Related Problems:
- Write a C program to remove duplicates from an unsorted linked list using a hash table.
- Write a C program to remove duplicate nodes from a singly linked list without using extra memory.
- Write a C program to remove duplicates only if a node appears more than once in the linked list.
- Write a C program to recursively remove duplicate nodes from an unsorted linked list.
C Programming Code Editor:
Previous: Check if a singly linked list is palindrome or not.
Next: Sort a singly linked list using merge sort.
What is the difficulty level of this exercise?