C Exercises: Insert new node at the end of a doubly linked list
C Doubly Linked List : Exercise-4 with Solution
Write a program in C to insert a new node at the end of a doubly linked list.
Visual Presentation:
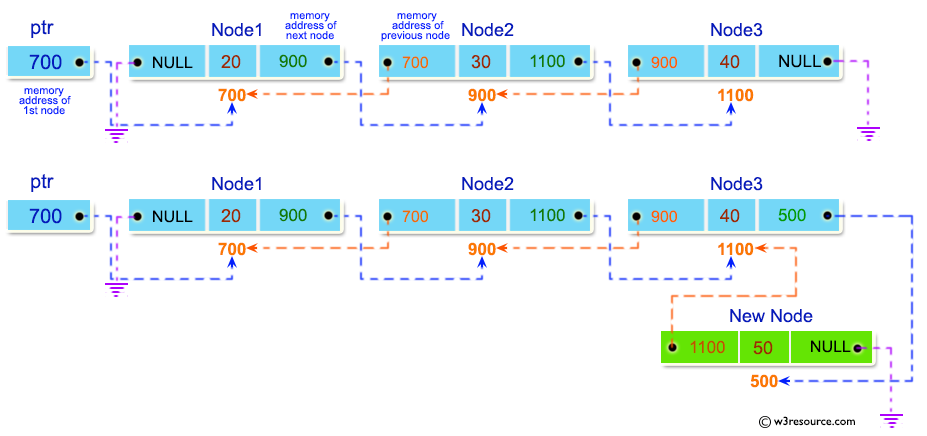
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Structure for doubly linked list node
struct node {
int num;
struct node *preptr;
struct node *nextptr;
} *stnode, *ennode;
// Function prototypes
void DlListcreation(int n);
void DlLinsertNodeAtEnd(int num);
void displayDlList(int a);
int main() {
int n, num1, a;
stnode = NULL;
ennode = NULL;
// User input for the number of nodes
printf("\n\n Doubly Linked List : Insert new node at the end in a doubly linked list :\n");
printf("------------------------------------------------------------------------------\n");
printf(" Input the number of nodes : ");
scanf("%d", &n);
// Creating the doubly linked list
DlListcreation(n);
a = 1;
displayDlList(a);
// Inserting a node at the end of the list
printf(" Input data for the last node : ");
scanf("%d", &num1);
DlLinsertNodeAtEnd(num1);
a = 2;
displayDlList(a);
return 0;
}
// Function to create a doubly linked list
void DlListcreation(int n) {
int i, num;
struct node *fnNode;
if (n >= 1) {
// Allocating memory for the first node
stnode = (struct node *)malloc(sizeof(struct node));
if (stnode != NULL) {
// Input data for the first node
printf(" Input data for node 1 : ");
scanf("%d", &num);
// Initializing values for the first node
stnode->num = num;
stnode->preptr = NULL;
stnode->nextptr = NULL;
ennode = stnode;
// Loop to create subsequent nodes and link them
for (i = 2; i <= n; i++) {
fnNode = (struct node *)malloc(sizeof(struct node));
if (fnNode != NULL) {
printf(" Input data for node %d : ", i);
scanf("%d", &num);
fnNode->num = num;
fnNode->preptr = ennode; // Linking new node with the previous node
fnNode->nextptr = NULL;
ennode->nextptr = fnNode; // Linking previous node with the new node
ennode = fnNode; // Assigning new node as the last node
} else {
printf(" Memory can not be allocated.");
break;
}
}
} else {
printf(" Memory can not be allocated.");
}
}
}
// Function to insert a node at the end of the doubly linked list
void DlLinsertNodeAtEnd(int num) {
struct node *newnode;
if (ennode == NULL) {
printf(" No data found in the list!\n");
} else {
// Allocating memory for the new node
newnode = (struct node *)malloc(sizeof(struct node));
newnode->num = num;
newnode->nextptr = NULL; // Setting next address field of new node as NULL
newnode->preptr = ennode; // Linking previous address of new node with the ending node
ennode->nextptr = newnode; // Linking next address of ending node with the new node
ennode = newnode; // Setting the new node as the ending node
}
}
// Function to display the doubly linked list
void displayDlList(int m) {
struct node *tmp;
int n = 1;
if (stnode == NULL) {
printf(" No data found in the List yet.");
} else {
tmp = stnode;
if (m == 1) {
printf("\n Data entered in the list are :\n");
} else {
printf("\n After insertion the new list are :\n");
}
// Loop to display nodes and their data
while (tmp != NULL) {
printf(" node %d : %d\n", n, tmp->num);
n++;
tmp = tmp->nextptr; // Moving the current pointer to the next node
}
}
}
Sample Output:
Doubly Linked List : Insert new node at the end in a doubly linked list : ------------------------------------------------------------------------------ Input the number of nodes : 3 Input data for node 1 : 2 Input data for node 2 : 5 Input data for node 3 : 8 Data entered in the list are : node 1 : 2 node 2 : 5 node 3 : 8 Input data for the last node : 9 After insertion the new list are : node 1 : 2 node 2 : 5 node 3 : 8 node 4 : 9
Flowchart:
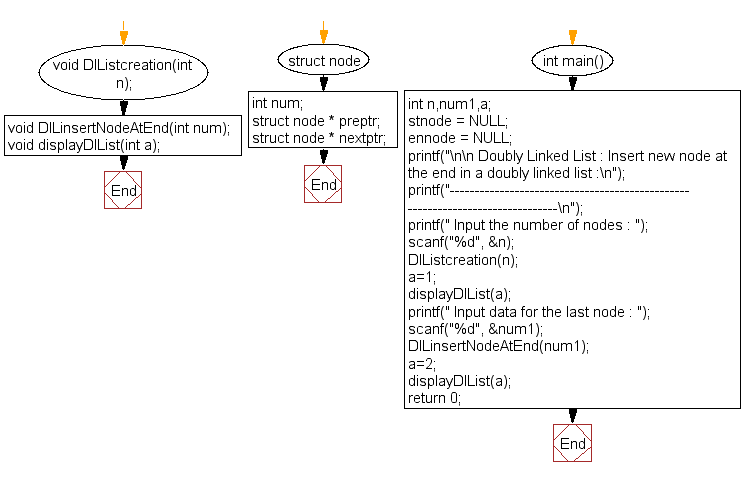
DlListcreation() :
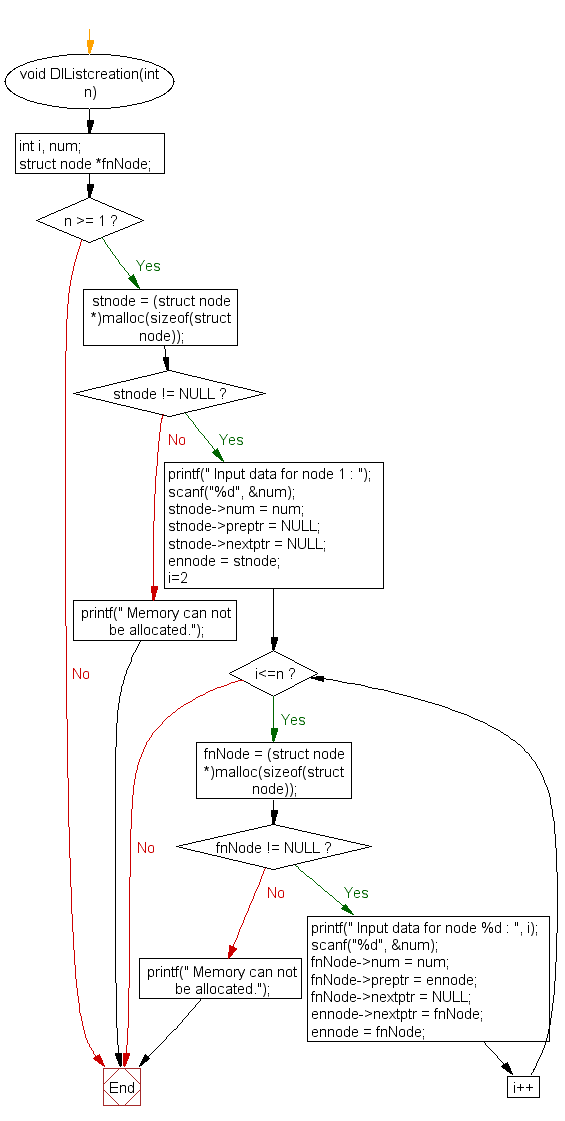
DlLinsertNodeAtEnd() :
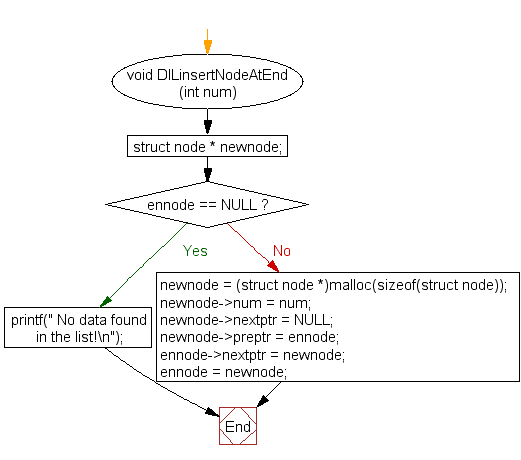
displayDlList() :
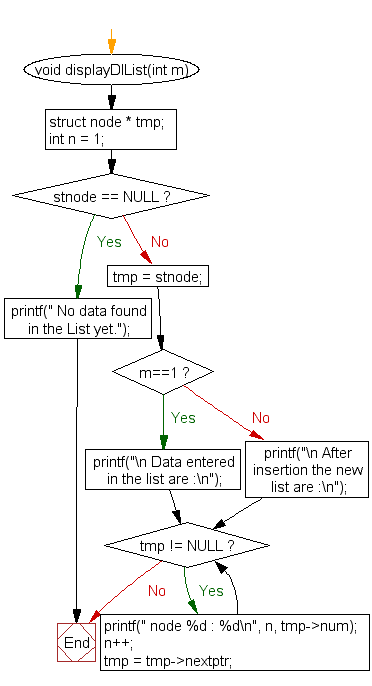
C Programming Code Editor:
Previous: Write a program in C to insert a new node at the beginning in a doubly linked list.
Next: Write a program in C to insert a new node at any position in a doubly linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics