C Exercises: Calculate the sum of three numbers getting input in one line
8. Sum of Three Numbers
Write a program in C to calculate the sum of three numbers with input on one line separated by a comma.
Pictorial Presentation:
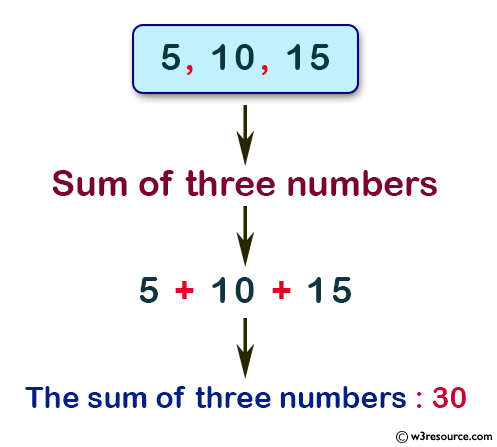
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
int num1, num2, num3; /* declaration of three variables */
int sum; /* variable to store the sum of numbers */
char line_text[50]; /* line of input from keyboard */
int main()
{
printf("Input three numbers separated by comma : "); // Prompt the user to input three numbers separated by comma.
fgets(line_text, sizeof(line_text), stdin); // Read a line of input from the user and store it in 'line_text'.
sscanf(line_text, "%d, %d, %d", &num1, &num2, &num3); // Convert the input to integers and store them in 'num1', 'num2', 'num3'.
sum = num1 + num2 + num3; // Calculate the sum of the three numbers.
printf("The sum of three numbers : %d\n", sum); // Print the sum of the three numbers.
return(0); // Return 0 to indicate successful execution of the program.
}
Sample Output:
Input three numbers seperated by comma : 5,10,15 The sum of three numbers : 30
Flowchart:
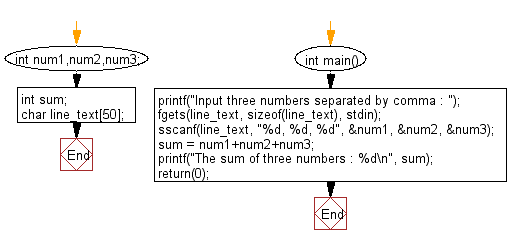
For more Practice: Solve these Related Problems:
- Write a C program to parse three comma-separated numbers from a single input line and calculate their product instead of the sum.
- Write a C program to compute the sum of three numbers provided in one line and then calculate their average.
- Write a C program to sum three comma-separated numbers and detect any non-numeric input, prompting for re-entry.
- Write a C program to sum three numbers provided on one line and then display them in ascending order.
C Programming Code Editor:
Previous: Write a program in C that reads a firstname, lastname and year of birth and display the names and the year one after another sequentially.
Next: Write a C program to perform addition, subtraction, multiplication and division of two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.