C Exercises: Calculates the total number of minutes with hour and minutes
C Input Output statement: Exercise-5 with Solution
Write a C program that takes hours and minutes as input, and calculates the total number of minutes.
Pictorial Presentation:
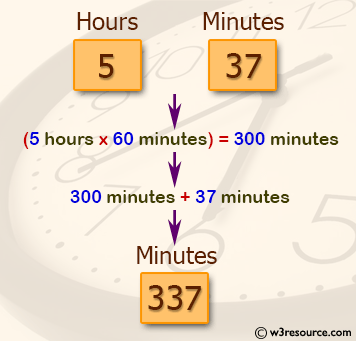
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
int hrs; /* given number of hours */
int mins; /* given number of minutes */
int tot_mins; /* total number of minutes (to be computed) */
const int MINaHOUR = 60; /* number of minutes in an hour */
char line_text[50]; /* line of input from keyboard */
int main() {
printf("Input hours: "); // Prompt the user to input hours.
fgets(line_text, sizeof(line_text), stdin); // Read a line of input from the user and store it in 'line_text'.
sscanf(line_text, "%d", &hrs); // Convert the input to an integer and store it in 'hrs'.
printf("Input minutes: "); // Prompt the user to input minutes.
fgets(line_text, sizeof(line_text), stdin); // Read a line of input from the user and store it in 'line_text'.
sscanf(line_text, "%d", &mins); // Convert the input to an integer and store it in 'mins'.
tot_mins = mins + (hrs * MINaHOUR); // Calculate the total number of minutes.
printf("Total: %d minutes.\n", tot_mins); // Print the total number of minutes.
return(0); // Return 0 to indicate successful execution of the program.
}
Sample Output:
Input hours: 5 Input minutes: 37 Total: 337 minutes.
Flowchart:
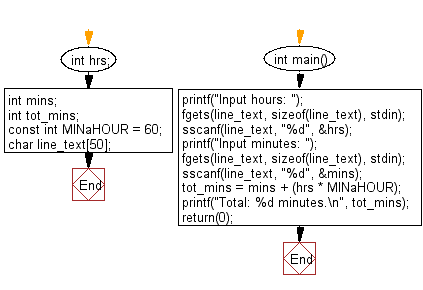
C Programming Code Editor:
Previous: Write a C program that converts kilometers per hour to miles per hour.
Next: Write a program in C that takes minutes as input, and display the total number of hours and minutes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/input-output/c-input-output-statement-exercises-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics