C Exercises: Converts kilometers per hour to miles per hour
Write a C program that converts kilometers per hour to miles per hour.
Pictorial Presentation:
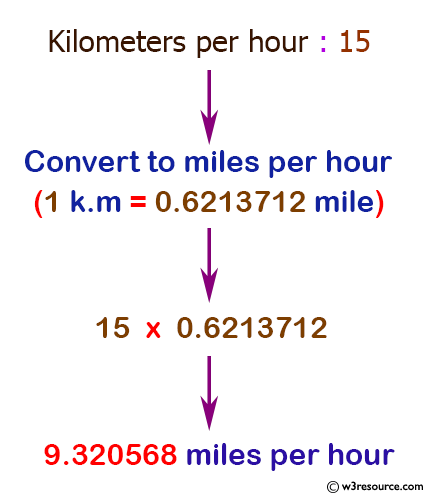
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
float kmph; /* kilometers per hour */
float miph; /* miles per hour (to be computed) */
char line_text[50]; /* a line from the keyboard */
int main()
{
printf("Input kilometers per hour: "); // Prompt the user to input kilometers per hour.
fgets(line_text, sizeof(line_text), stdin); // Read a line of input from the user and store it in 'line_text'.
sscanf(line_text, "%f", &kmph); // Convert the input to a float and store it in 'kmph'.
miph = (kmph * 0.6213712); // Convert kilometers per hour to miles per hour.
printf("%f miles per hour\n", miph); // Print the result in miles per hour.
return(0); // Return 0 to indicate successful execution of the program.
}
Sample Output:
Input kilometers per hour: 15 9.320568 miles per hour
Flowchart:
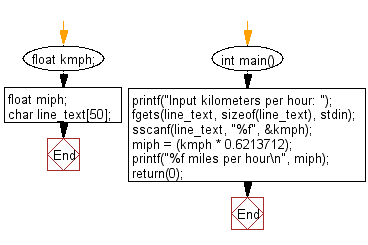
C Programming Code Editor:
Previous: Write a C program that prints the perimeter of a rectangle to take its height and width as input.
Next: Write a C program that takes hours and minutes as input, and calculates the total number of minutes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics