C Exercises: Calculate the perimeter of a rectangle
3. Rectangle Perimeter Calculation
Write a C program that prints the perimeter of a rectangle using its height and width as inputs.
Pictorial Presentation:
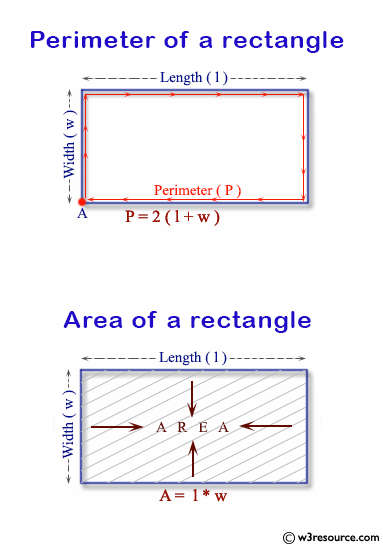
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
int main() {
float rec_width; /* Declare variable 'rec_width' to store the width of the rectangle */
float rec_height; /* Declare variable 'rec_height' to store the height of the rectangle */
float rec_perimeter; /* Declare variable 'rec_perimeter' to store the perimeter (to be computed) */
// Prompt the user to input the height of the rectangle.
printf("Input the height of the Rectangle : ");
scanf("%f", &rec_height); // Read the value of 'rec_height' from the user.
// Prompt the user to input the width of the rectangle.
printf("Input the width of the Rectangle : ");
scanf("%f", &rec_width); // Read the value of 'rec_width' from the user.
// Calculate the perimeter of the rectangle using the formula: perimeter = 2 * ( width + height )
rec_perimeter = 2.0 * (rec_height + rec_width);
// Print the calculated perimeter of the rectangle.
printf("Perimeter of the Rectangle is : %f\n", rec_perimeter);
return 0; // Indicate successful program execution.
}
Sample Output:
Input the height of the Rectangle : 5 Input the width of the Rectangle : 7 Perimeter of the Rectangle is : 24.000000
Flowchart:
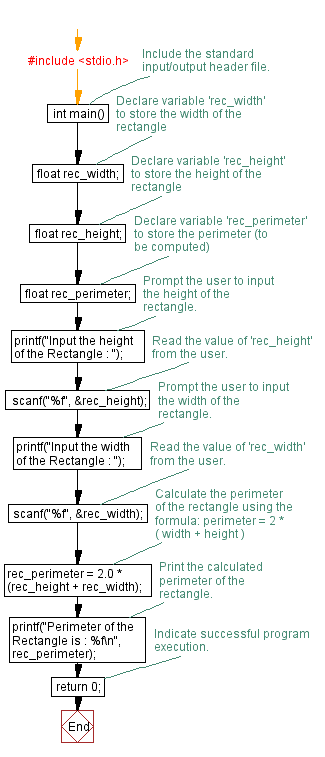
For more Practice: Solve these Related Problems:
- Write a C program to calculate the perimeter of a rectangle and then compute the difference between its perimeter and area.
- Write a C program to compute the perimeter of a rectangle with height and width provided as command-line arguments.
- Write a C program to calculate the perimeter of a rectangle and ensure that both height and width inputs are positive numbers.
- Write a C program to read dimensions for multiple rectangles from a file and output the largest perimeter.
C Programming Code Editor:
Previous: Write a C program that calculates the volume of a sphere.
Next: Write a C program that converts kilometers per hour to miles per hour.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics