C Exercises: Calculates the volume of a sphere
2. Volume of a Sphere Calculation
Write a C program that calculates the volume of a sphere.
C Programming : Volume of a sphere
A sphere is a perfectly round geometrical object in three-dimensional space that is the surface of a completely round ball.
In three dimensions, the volume inside a sphere is derived to be V = 4/3*π*r3 where r is the radius of the sphere
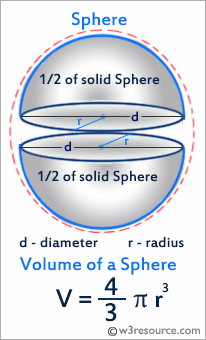
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
float myradius; /* radius of the sphere */
float myvolume; /* volume of the sphere (to be computed) */
char line_text[50]; /* a line from the keyboard */
/* the value of pi to 50 places, from wikipedia */
const float PI = 3.14159265358979323846264338327950288419716939937510;
int main() {
printf("Input the radius of the sphere : "); // Prompt the user to input the radius of the sphere.
fgets(line_text, sizeof(line_text), stdin); // Read a line of input from the user and store it in 'line_text'.
sscanf(line_text, "%f", &myradius); // Convert the input from 'line_text' to a float and store it in 'myradius'.
myvolume = (4.0 / 3.0) * PI * (myradius * myradius * myradius); /* Calculate the volume of the sphere using the formula. */
printf("The volume of sphere is %f.\n", myvolume); // Print the calculated volume of the sphere.
return(0); // Return 0 to indicate successful execution of the program.
}
Sample Output:
Input the radius of the sphere : 2.56 The volume of sphere is 70.276237.
Flowchart:
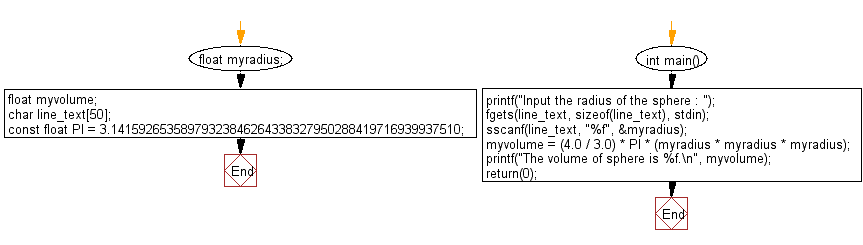
For more Practice: Solve these Related Problems:
- Write a C program to calculate the volume of a sphere using the power function and compare it with a hardcoded approximation of Pi.
- Write a C program to compute the volume of a sphere given its diameter and handle floating-point precision issues.
- Write a C program to calculate the volume of a sphere and then determine the difference in volume between two spheres with consecutive radii.
- Write a C program to calculate the volume of a sphere and validate the input radius to ensure it is positive.
C Programming Code Editor:
Previous: Write a C program that convert a temperature from Centigrade to Fahrenheit.
Next: Write a C program that prints the perimeter of a rectangle to take its height and width as input.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics