C Exercises: Check if a given integer is even or odd using an inline function
Write a C program to check if a given integer is even or odd using an inline function.
Sample Solution:
C Code:
#include <stdio.h>
inline int isEven(int n) {
return n % 2 == 0;
}
int main() {
int num;
printf("Input an integer: ");
scanf("%d", & num);
if (isEven(num)) {
printf("%d is even.\n", num);
} else {
printf("%d is odd.\n", num);
}
return 0;
}
Sample Output:
Input an integer: 3 3 is odd. Input an integer: 46 46 is even.
Explanation:
In the above program we first define an inline function isEven() that takes an integer as an argument and returns a boolean indicating whether the integer is even or not. The main function then prompts the user to input an integer, passes it to the isEven() function, and prints a message indicating whether the integer is even or odd.
Flowchart:
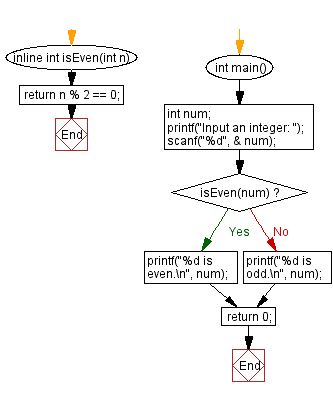
C Programming Code Editor:
Previous: Check if a given integer is prime using an inline function.
Next: Reverse a given string using an inline function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics