C Exercises: Count the number of vowels in a given string using an inline function
7. Count Vowels in String Inline Variants
Write a C program to count the number of vowels in a given string using an inline function.
Sample Solution:
C Code:
#include <stdio.h>
#include <ctype.h>
inline int count_vowels(const char * str) {
int ctr = 0;
while ( * str != '\0') {
char ch = tolower( * str);
if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u') {
ctr++;
}
str++;
}
return ctr;
}
int main() {
char str[] = "w3resource.com";
int vowels = count_vowels(str);
printf("The string \"%s\" contains %d vowels.\n", str, vowels);
char str1[] = "United States of America ";
vowels = count_vowels(str1);
printf("\nThe string \"%s\" contains %d vowels.\n", str1, vowels);
return 0;
}
Sample Output:
The string "w3resource.com" contains 5 vowels. The string "United States of America " contains 10 vowels.
Explanation:
The above count_vowels() function uses a while loop to iterate over each character in the string. It converts each character to lowercase using the tolower() function and then checks if it is a vowel (i.e., 'a', 'e', 'i', 'o', or 'u'). If the character is a vowel, it increments a counter variable. Finally, the function returns the total count of vowels in the string.
In the main() function, we declare a test string, call the count_vowels() function on it, and print the result.
Flowchart:
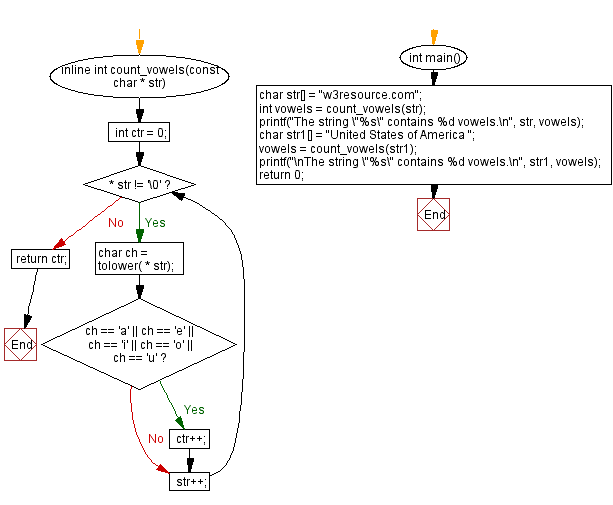
For more Practice: Solve these Related Problems:
- Write a C program to count the number of consonants in a given string using an inline function.
- Write a C program to count vowels in a string ignoring case using an inline function.
- Write a C program to count vowels and output their frequency distribution using an inline function.
- Write a C program to count vowels from multiple strings passed to an inline function and sum the counts.
C Programming Code Editor:
Previous: Convert a temperature from Celsius to Fahrenheit and vice versa.
Next: Check if a given integer is prime using an inline function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.