C Exercises: Compute the sum of a variable number of integers passed as arguments using an inline function
C inline function: Exercise-11 with Solution
Write a C program to compute the sum of a variable number of integers passed as arguments using an inline function.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdarg.h>
inline int inline_sum(int count, ...) {
int sum = 0;
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
sum += va_arg(args, int);
}
va_end(args);
return sum;
}
int main() {
int total = inline_sum(5, 1, 2, 3, 4, 5);
printf("Sum is %d\n", total);
int total2 = inline_sum(3, 6, 10, -16);
printf("Sum is %d\n", total2);
return 0;
}
Sample Output:
Sum is 15 Sum is 0
Explanation:
In the above exercise, the sum function takes a variable number of arguments using the stdarg.h library. It first initializes a va_list object with va_start, then iterates through the arguments in a loop. It adds each one to a running sum. Finally, it cleans up with va_end and returns the sum.
In main(), we call sum twice with different sets of integers and print out the results.
Flowchart:
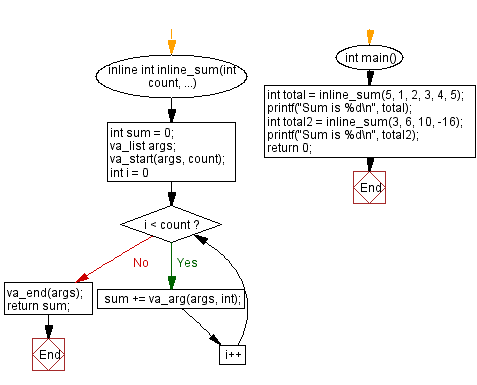
C Programming Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/inline_function/c-inline-function-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics