C Exercises: Find the sum of specified series
5. Series Sum Using Function Variants
Write a program in C to find the sum of the series 1!/1+2!/2+3!/3+4!/4+5!/5 using the function.
Pictorial Presentation:
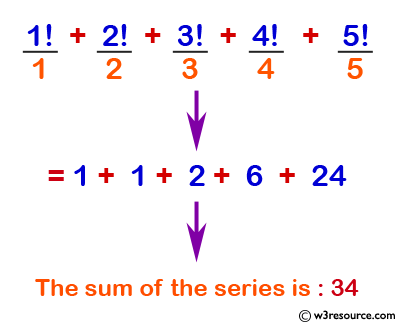
Sample Solution:
C Code:
#include <stdio.h>
int fact(int);
void main()
{
int sum;
sum=fact(1)/1+fact(2)/2+fact(3)/3+fact(4)/4+fact(5)/5;
printf("\n\n Function : find the sum of 1!/1+2!/2+3!/3+4!/4+5!/5 :\n");
printf("----------------------------------------------------------\n");
printf("The sum of the series is : %d\n\n",sum);
}
int fact(int n)
{
int num=0,f=1;
while(num<=n-1)
{
f =f+f*num;
num++;
}
return f;
}
Sample Output:
Function : find the sum of 1!/1+2!/2+3!/3+4!/4+5!/5 : ---------------------------------------------------------- The sum of the series is : 34
Explanation:
int fact(int n) { int num = 0, f = 1; while (num <= n - 1) { f = f + f * num; num++; } return f; }
In the above code the function 'fact' takes a single argument 'n' (type int). It computes the factorial of the input number 'n' using a while loop. The function initializes two local integer variables, 'num' and 'f', to 0 and 1 respectively. It then enters a loop that iterates n-1 times, incrementing num by 1 in each iteration. In each iteration of the loop, the value of f is updated by multiplying it with num incremented by 1. This computes the factorial of 'n' and returns the result.
Time complexity and space complexity:
The time complexity of the function 'fact' is O(n), where 'n' is the input number, as the loop in the function iterates n-1 times.
The space complexity of this function is O(1), as it only uses a fixed amount of memory to store the two integer variables 'num' and 'f'.
Flowchart:
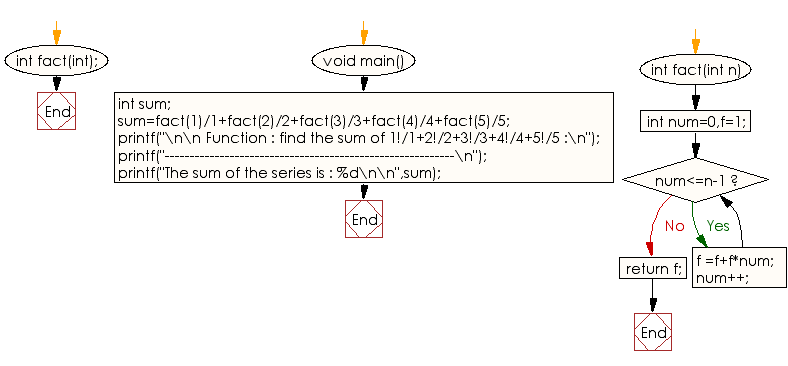
For more Practice: Solve these Related Problems:
- Write a C program to calculate the sum of the series n!/n for n from 1 to a given number using a dedicated function.
- Write a C program to compute the sum of a modified series where each term is n! divided by (n+1) using a function.
- Write a C program that uses recursion within a function to calculate each term of the series and sums them.
- Write a C program to compute the series sum 1/1! + 2/2! + 3/3! + ... and compare the result with the expected mathematical constant.
C Programming Code Editor:
Previous: Write a program in C to check a given number is even or odd using the function.
Next: Write a program in C to convert decimal number to binary number using the function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.