C Exercises: Check whether two given strings are an anagram
11. Anagram Check Function Variants
Write a program in C to check whether two given strings are an anagram.
Pictorial Presentation:
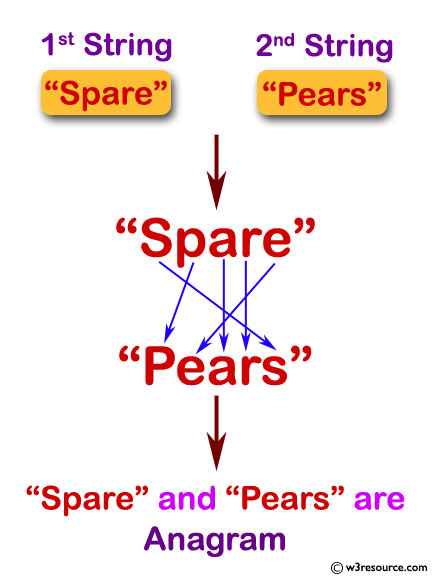
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
//Two strings are anagram of each other, if we can rearrange
//characters of one string to form another string. All the characters
//of one string must be present in another string and should appear same
//number of time in other string. Strings can contain any ASCII characters.
//Example : rescued and secured, resign and singer, stone and tones,
//pears and spare, ELEVEN PLUS TWO and TWELVE PLUS ONE
int checkAnagram(char *str1, char *str2);
int main()
{
char str1[100], str2[100];
printf("\n\n Function : whether two given strings are anagram :\n");
printf("\n\n Example : pears and spare, stone and tones :\n");
printf("-------------------------------------------------------\n");
printf(" Input the first String : ");
fgets(str1, sizeof str1, stdin);
printf(" Input the second String : ");
fgets(str2, sizeof str2, stdin);
if(checkAnagram(str1, str2) == 1)
{
str1[strlen(str1)-1] = '\0';
str2[strlen(str2)-1] = '\0';
printf(" %s and %s are Anagram.\n\n",str1,str2);
}
else
{
str1[strlen(str1)-1] = '\0';
str2[strlen(str2)-1] = '\0';
printf(" %s and %s are not Anagram.\n\n",str1,str2);
}
return 0;
}
//Function to check whether two passed strings are anagram or not
int checkAnagram(char *str1, char *str2)
{
int str1ChrCtr[256] = {0}, str2ChrCtr[256] = {0};
int ctr;
/* check the length of equality of Two Strings */
if(strlen(str1) != strlen(str2))
{
return 0;
}
//count frequency of characters in str1
for(ctr = 0; str1[ctr] != '\0'; ctr++)
{
str1ChrCtr[str1[ctr]]++;
}
//count frequency of characters in str2
for(ctr = 0; str2[ctr] != '\0'; ctr++)
{
str2ChrCtr[str2[ctr]]++;
}
//compare character counts of both strings
for(ctr = 0; ctr < 256; ctr++)
{
if(str1ChrCtr[ctr] != str2ChrCtr[ctr])
return 0;
}
return 1;
}
Sample Output:
Function : whether two given strings are anagram : Example : pears and spare, stone and tones : ------------------------------------------------------- Input the first String : spare Input the second String : pears spare and pears are Anagram.
Explanation:
int checkAnagram(char * str1, char * str2) { int str1ChrCtr[256] = { 0 }, str2ChrCtr[256] = { 0 }; int ctr; /* check the length of equality of Two Strings */ if (strlen(str1) != strlen(str2)) { return 0; } //count frequency of characters in str1 for (ctr = 0; str1[ctr] != '\0'; ctr++) { str1ChrCtr[str1[ctr]]++; } //count frequency of characters in str2 for (ctr = 0; str2[ctr] != '\0'; ctr++) { str2ChrCtr[str2[ctr]]++; } //compare character counts of both strings for (ctr = 0; ctr < 256; ctr++) { if (str1ChrCtr[ctr] != str2ChrCtr[ctr]) return 0; } return 1; }
The 'checkAnagram' function takes two arguments 'str1' and 'str2' of type char. It checks whether the two input strings are anagrams of each other or not. The function initializes two integer arrays, 'str1ChrCtr' and 'str2ChrCtr', of size 256, which correspond to the number of possible ASCII characters. It then enters a conditional statement to check if the lengths of ‘str1’ and ‘str2’ are equal, and returns 0 if they are not equal.
The function then iterates through 'str1' and 'str2' separately, counting the frequency of each character in the strings by incrementing the corresponding entry in the 'str1ChrCtr' and 'str2ChrCtr' arrays. Finally, the function compares the two arrays, character by character, and returns 0 if any entry in the arrays differs. If all entries in the arrays match, then the function returns 1, indicating that the two input strings are anagrams of each other.
Time complexity and space complexity:
The time complexity of this function is O(n), where n is the length of the input strings str1 and str2, because the function iterates through each character of the two input strings and each iteration takes constant time. The space complexity of this function is O(1), because the size of the two integer arrays ‘str1ChrCtr’ and 'str2ChrCtr' is constant and independent of the input size.
Flowchart:
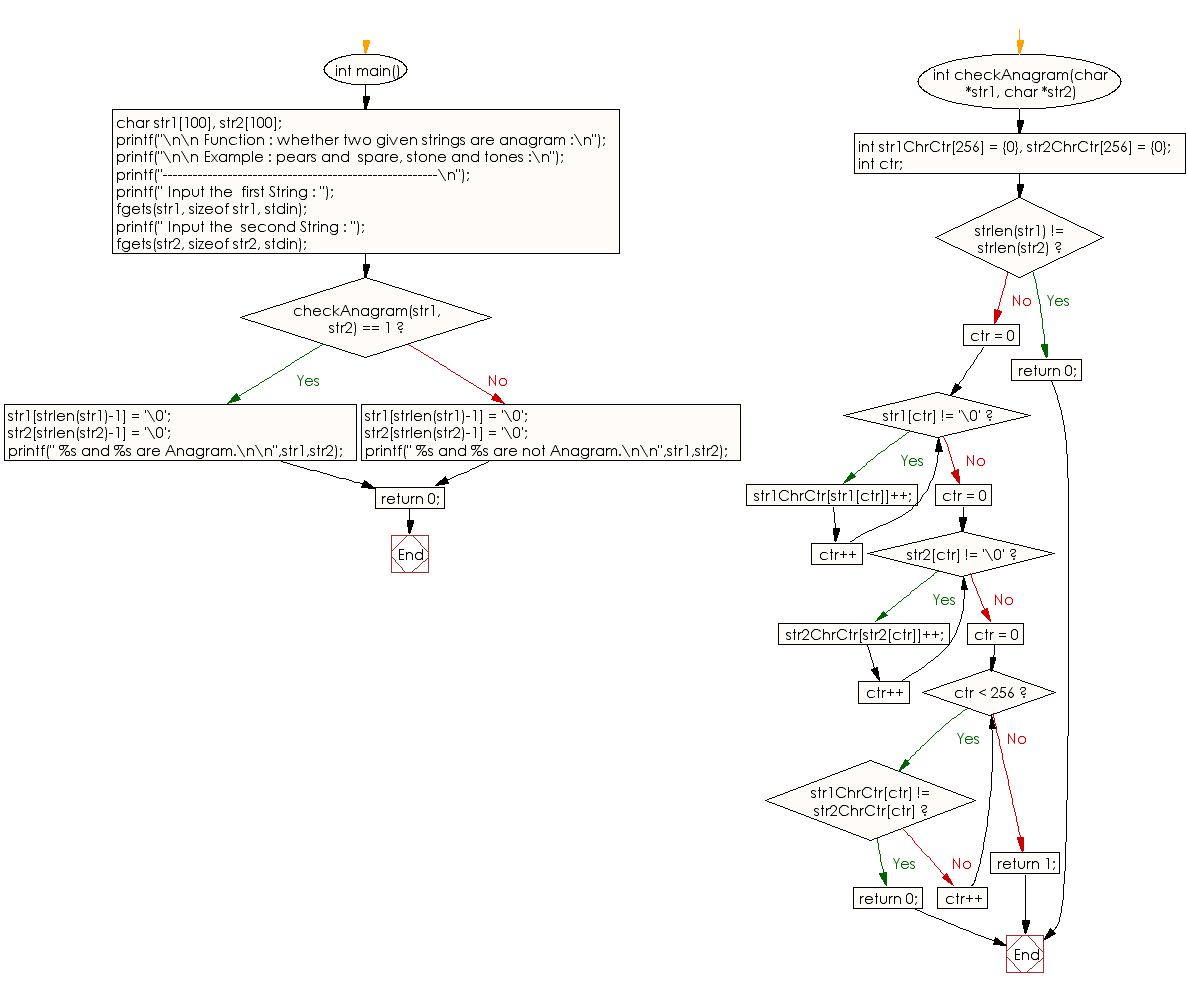
For more Practice: Solve these Related Problems:
- Write a C program to check if two strings are anagrams by sorting their characters in a function.
- Write a C program to compare the frequency of characters in two strings using a function and determine anagram status.
- Write a C program to verify anagrams by ignoring case and whitespace through a dedicated function.
- Write a C program to return a boolean value from a function that checks if two strings are anagrams.
C Programming Code Editor:
Previous: Write a program in C to print all perfect numbers in given range using the function.
Next: Write a C programming to find out maximum and minimum of some values using function which will return an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.