C Exercises: Display n number of multiplication table vertically
7. Vertical Multiplier Table from 1 to n
Write a program in C to display the multiplier table vertically from 1 to n.
This C program generates and displays the multiplication table vertically from 1 to a user-specified integer n. The user inputs an integer, and the program uses nested loops to iterate through numbers 1 to 10 for each multiplier from 1 to nnn. Each column represents a multiplier, and the rows show the product of the multiplier with numbers 1 to 10.
Visual Presentation:
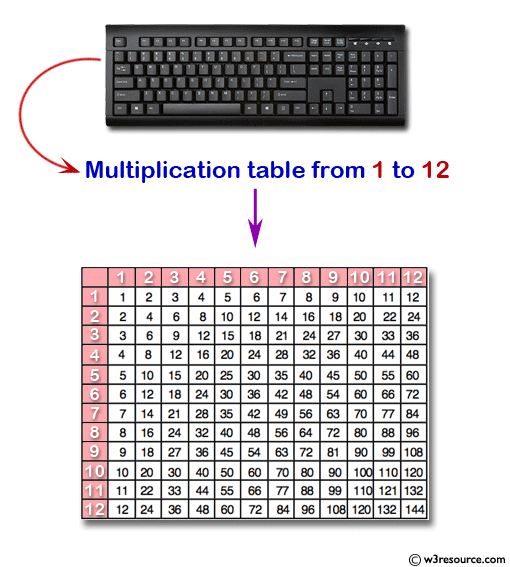
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
int main() {
int j, i, n; // Declare variables 'j' and 'i' for loop counters, and 'n' for user input.
printf("Input upto the table number starting from 1 : "); // Print a message to prompt user input.
scanf("%d", &n); // Read the value of 'n' from the user.
printf("Multiplication table from 1 to %d \n", n); // Print a message showing the range of the table.
for (i = 1; i <= 10; i++) { // Start an outer loop for the numbers 1 to 10.
for (j = 1; j <= n; j++) { // Start an inner loop for the table range.
printf("%dx%d = %d, ", j, i, i * j); // Print the multiplication expression and result.
}
printf("\n"); // Print a newline to move to the next row of the table.
}
return 0; // Indicate that the program has executed successfully.
}
Output:
Input upto the table number starting from 1 : 8 Multiplication table from 1 to 8 1x1 = 1, 2x1 = 2, 3x1 = 3, 4x1 = 4, 5x1 = 5, 6x1 = 6, 7x1 = 7, 8x1 = 8 1x2 = 2, 2x2 = 4, 3x2 = 6, 4x2 = 8, 5x2 = 10, 6x2 = 12, 7x2 = 14, 8x2 = 16 1x3 = 3, 2x3 = 6, 3x3 = 9, 4x3 = 12, 5x3 = 15, 6x3 = 18, 7x3 = 21, 8x3 = 24 1x4 = 4, 2x4 = 8, 3x4 = 12, 4x4 = 16, 5x4 = 20, 6x4 = 24, 7x4 = 28, 8x4 = 32 1x5 = 5, 2x5 = 10, 3x5 = 15, 4x5 = 20, 5x5 = 25, 6x5 = 30, 7x5 = 35, 8x5 = 40 1x6 = 6, 2x6 = 12, 3x6 = 18, 4x6 = 24, 5x6 = 30, 6x6 = 36, 7x6 = 42, 8x6 = 48 1x7 = 7, 2x7 = 14, 3x7 = 21, 4x7 = 28, 5x7 = 35, 6x7 = 42, 7x7 = 49, 8x7 = 56 1x8 = 8, 2x8 = 16, 3x8 = 24, 4x8 = 32, 5x8 = 40, 6x8 = 48, 7x8 = 56, 8x8 = 64 1x9 = 9, 2x9 = 18, 3x9 = 27, 4x9 = 36, 5x9 = 45, 6x9 = 54, 7x9 = 63, 8x9 = 72 1x10 = 10, 2x10 = 20, 3x10 = 30, 4x10 = 40, 5x10 = 50, 6x10 = 60, 7x10 = 70, 8x10 = 80
Explanation:
for (i = 1; i <= 10; i++) { for (j = 1; j <= n; j++) { printf("%dx%d = %d, ", j, i, i * j); } printf("\n"); }
In this loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to 10. In each iteration of the outer loop, another loop is started with variable j, initialized to 1, and it will continue as long as j is less than or equal to the value of variable 'n'.
In each iteration of the inner loop, the printf function will print a formatted string to the console. The string will display the value of j, the value of i, and the product of i and j (i.e., ij). The placeholders %d will be replaced by the values of j, i, and ij, respectively. Additionally, the string will include a comma and space character (", ") after each product.
After the inner loop completes, the outer loop will print a newline character (\n) to create a new line.
Finally, the outer loop will increment the value of i by 1, and the process will repeat until the condition i<=10 is no longer true.
Flowchart
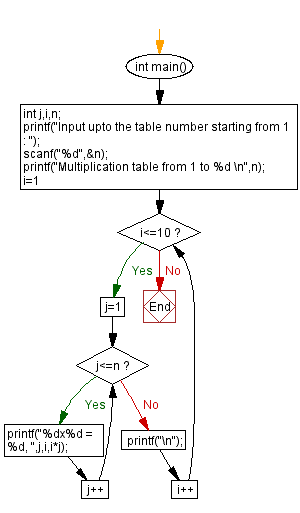
For more Practice: Solve these Related Problems:
- Write a C program to display a vertical multiplier table for numbers 1 to n using nested loops.
- Write a C program to print a vertical multiplier table for numbers 1 to n in reverse order.
- Write a C program to display a vertical multiplier table with each column’s sum calculated at the end.
- Write a C program to generate a vertical multiplier table for 1 to n and format the output into neatly aligned columns.
C Programming Code Editor:
Previous: Write a program in C to display the multiplication table of a given integer.
Next: Write a program in C to display the n terms of odd natural number and their sum like:
1 3 5 7 ... n
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.