C Exercises: Compute multiplication table of a given integer
6. Multiplication Table for a Given Integer
Write a program in C to display the multiplication table for a given integer.
This C program generates and displays the multiplication table for a specified integer. The user inputs an integer, and the program uses a "for" loop to iterate through numbers 1 to 10 (or any specified range), multiplying the input integer by each of these numbers. The results are printed in a formatted table, demonstrating basic loops and arithmetic operations in C.
Visual Presentation:
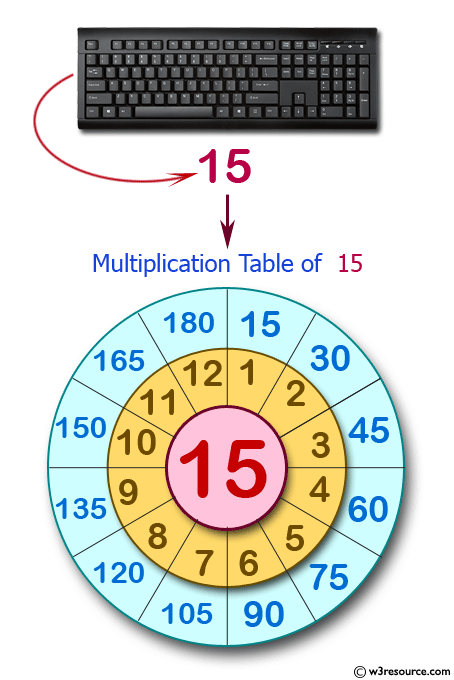
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int j, n; // Declare variables 'j' for loop counter and 'n' for user input.
printf("Input the number (Table to be calculated) : "); // Print a message to prompt user input.
scanf("%d", &n); // Read the value of 'n' from the user.
printf("\n"); // Print a newline for formatting.
for (j = 1; j <= 10; j++) { // Start a for loop to calculate the table up to 10.
printf("%d X %d = %d \n", n, j, n * j); // Print the multiplication expression and result.
}
}
Output:
Input the number (Table to be calculated) : 15 15 X 1 = 15 15 X 2 = 30 15 X 3 = 45 15 X 4 = 60 15 X 5 = 75 15 X 6 = 90 15 X 7 = 105 15 X 8 = 120 15 X 9 = 135 15 X 10 = 150
Explanation:
for (j = 1; j <= 10; j++) { printf("%d X %d = %d \n", n, j, n * j); }
In the above for loop, the variable j is initialized to 1, and the loop will continue as long as j is less than or equal to 10. In each iteration of the loop, the printf function will print a formatted string to the console.
The string will display the value of n, the value of j, and the product of n and j (i.e., nj). The placeholders %d will be replaced by the values of n, j, and nj, respectively. Additionally, the string will include a newline character (\n) to create a new line after each product.
The loop will increment the value of j by 1, and the process will repeat until the condition j<=10 is no longer true.
Flowchart:
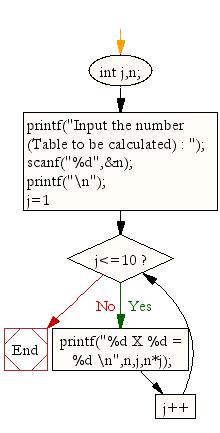
For more Practice: Solve these Related Problems:
- Write a C program to display the multiplication table for a given integer in reverse order.
- Write a C program to print the multiplication table for a given integer while skipping multiples of a specified number.
- Write a C program to display the multiplication table for a given integer using recursion.
- Write a C program to format and display the multiplication table for a given integer with proper column alignment.
C Programming Code Editor:
Previous: Write a program in C to display the cube of the number upto a given integer.
Next: Write a program in C to display the multiplication table vertically from 1 to n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.