C Exercises: Convert decimal number to octal without using an array
50. Decimal to Octal Conversion (Without Array)
Write a program in C to convert a decimal number into octal without using an array.
This C program converts a decimal number into octal without using an array. It initializes variables for the decimal number and a multiplier for octal digits. Then, it iterates through the decimal number, calculating each octal digit and updating the result accordingly. Finally, it prints the converted octal number.
Visual Presentation:
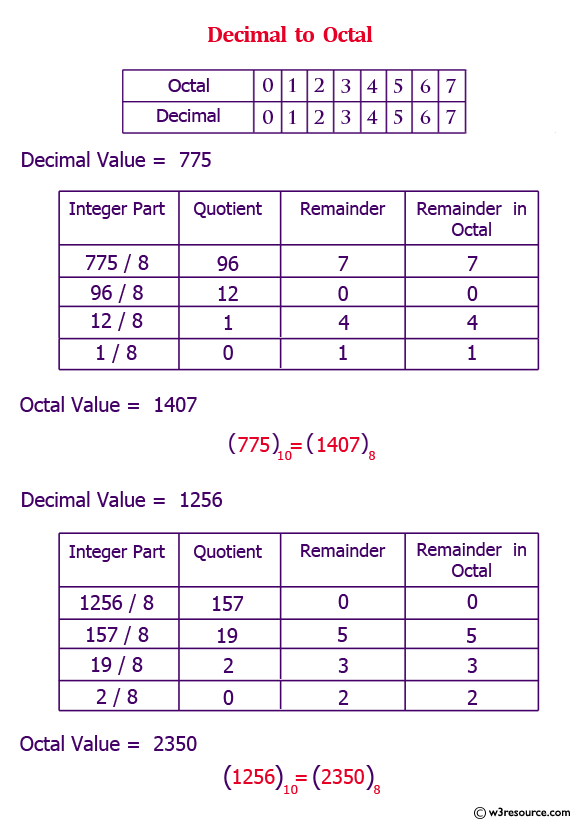
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int n, i, j, ocno = 0, dn; // Declare variables to store input and results.
printf("\n\nConvert Decimal to Octal:\n "); // Print a message.
printf("-------------------------\n"); // Print a separator.
printf("Enter a number to convert : "); // Prompt the user for input.
scanf("%d", &n); // Read the input decimal number.
dn = n; // Store the original value of the decimal number.
i = 1; // Initialize the multiplier for the octal digits.
for (j = n; j > 0; j = j / 8) {
ocno = ocno + (j % 8) * i; // Calculate the octal digit and add it to the result.
i = i * 10; // Update the multiplier for the next octal digit.
n = n / 8; // Update the quotient for the next iteration.
}
printf("\nThe Octal of %d is %d.\n\n", dn, ocno); // Print the result.
}
Output:
Convert Decimal to Octal: ------------------------- Enter a number to convert : 79 The Octal of 79 is 117.
Flowchart:
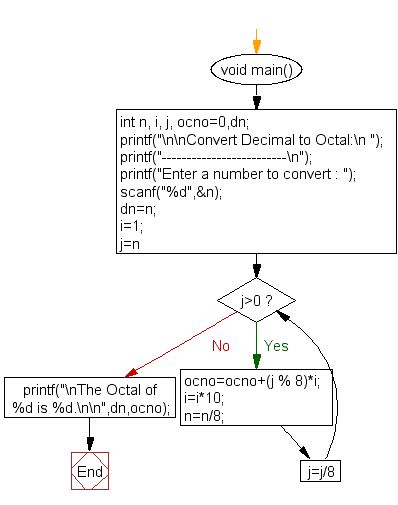
For more Practice: Solve these Related Problems:
- Write a C program to convert a decimal number to octal without using arrays by applying recursion.
- Write a C program to convert a decimal number to octal without arrays and count the number of octal digits.
- Write a C program to convert a decimal number to octal without arrays and display the output in a formatted string.
- Write a C program to convert a decimal number to octal without arrays and verify the conversion by converting it back.
C Programming Code Editor:
Previous: Write a c program to find out the sum of an A.P. series.
Next: Write a program in C to convert a Octal number to a Decimal number without using an array, function and while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.