C Exercises: Display the number in reverse order
37. Reverse a Given Number
Write a program in C to display a given number in reverse order.
The program should prompt the user to input a number, then process the number to reverse its digits. Finally, the program will display the reversed number to the user.
Visual Presentation:
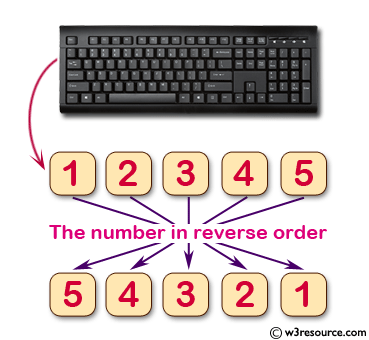
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main(){
int num, r, sum = 0, t; // Declare variables for the original number, remainder, reversed number, and temporary variable.
printf("Input a number: "); // Prompt the user to input a number.
scanf("%d", &num); // Read the input from the user.
for(t = num; num != 0; num = num / 10){ // Loop to reverse the digits of the number.
r = num % 10; // Extract the last digit (remainder when divided by 10).
sum = sum * 10 + r; // Build the reversed number by adding the extracted digit.
}
printf("The number in reverse order is : %d \n", sum); // Print the reversed number.
}
Output:
Input a number: 12345 The number in reverse order is : 54321
Flowchart:
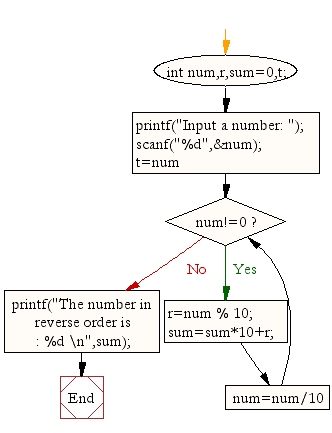
For more Practice: Solve these Related Problems:
- Write a C program to reverse a number using recursion instead of loops.
- Write a C program to reverse a number without using division or modulus operators (convert to string method).
- Write a C program to reverse the digits of a number and then check if the original and reversed numbers are identical (palindrome check).
- Write a C program to reverse a number and then output the difference between the original number and its reverse.
C Programming Code Editor:
Previous: Write a program in C to display the such a pattern for n number of rows using a number which will start with the number 1 and the first and a last number of each row will be 1.
Next: Write a program in C to check whether a number is a palindrome or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.