C Exercises: Print the Floyd's Triangle
C For Loop: Exercise-22 with Solution
Write a program in C to print Floyd's Triangle.
The Floyd's triangle is as below :
1 01 101 0101 10101
This C program prints Floyd's Triangle, a triangular array of binary numbers (0s and 1s). The program prompts the user for the number of rows and then generates the triangle by alternating between 0s and 1s in a specific pattern. The resulting triangle is printed row by row, creating a visually distinct pattern with each row having an increasing number of elements.
Visual Presentation:
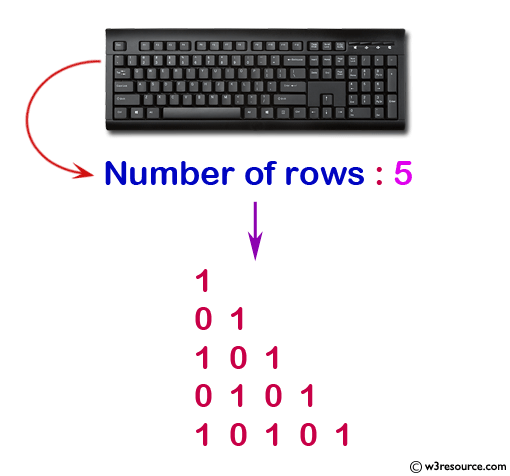
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, j, n, p, q; // Declare variables to store input and control loop indices.
printf("Input number of rows : "); // Prompt the user for input.
scanf("%d", &n); // Read the value of 'n' from the user.
for (i = 1; i <= n; i++) // Loop for the number of rows.
{
if (i % 2 == 0) // Check if 'i' is even.
{
p = 1;
q = 0;
}
else // If 'i' is odd.
{
p = 0;
q = 1;
}
for (j = 1; j <= i; j++) // Loop for each element in the row.
{
if (j % 2 == 0) // Check if 'j' is even.
printf("%d", p); // Print 'p' if 'j' is even.
else
printf("%d", q); // Print 'q' if 'j' is odd.
}
printf("\n"); // Move to the next line after printing a row.
}
}
Output:
Input number of rows : 5 1 01 101 0101 10101
Flowchart:
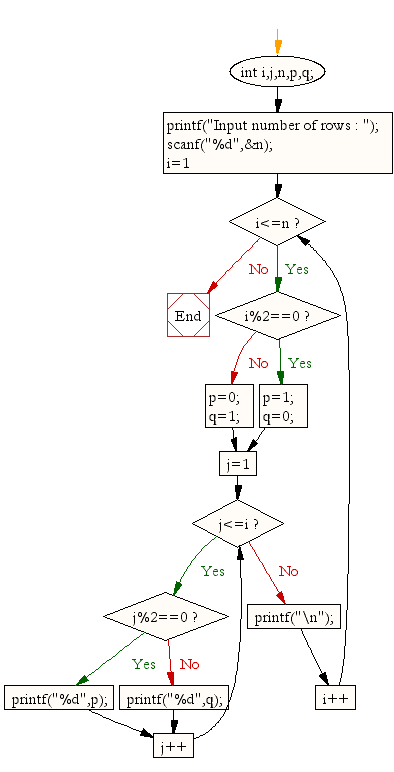
C Programming Code Editor:
Previous: Write a program in C to display the sum of the series [ 9 + 99 + 999 + 9999 ...].
Next: Write a program in C to display the sum of the series [ 1+x+x^2/2!+x^3/3!+....].
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/for-loop/c-for-loop-exercises-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics