C Exercises: Calculate the harmonic series and their sum
Write a program in C to display the n terms of a harmonic series and their sum.
The series is : 1 + 1/2 + 1/3 + 1/4 + 1/5 ... 1/n terms
This C program displays the first n terms of a harmonic series and calculates their sum. The harmonic series is represented as 1 + 1/2 + 1/3 + 1/4 + 1/5 ... 1/n. The program will prompt the user to input the value of n, then use a loop to compute and display each term, as well as the cumulative sum of the series.
Visual Presentation:
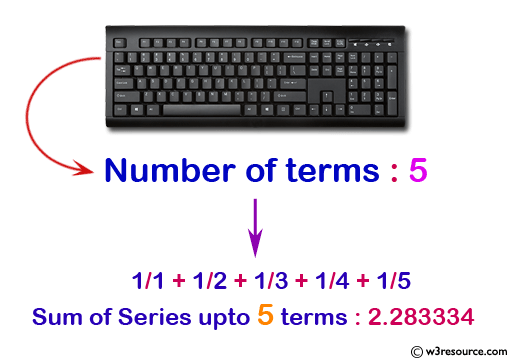
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, n; // Declare variables to store input and loop control.
float s = 0.0; // Initialize 's' to 0.0 to accumulate the sum.
// Prompt the user to input the number of terms.
printf("Input the number of terms : ");
scanf("%d", &n); // Read the value of 'n' from the user.
printf("\n\n"); // Print extra new lines for formatting.
// Loop to calculate the sum of the series.
for (i = 1; i <= n; i++)
{
if (i < n)
{
printf("1/%d + ", i); // Print the term with a plus sign.
s += 1 / (float)i; // Calculate and add the term to the sum.
}
if (i == n)
{
printf("1/%d ", i); // Print the last term without a plus sign.
s += 1 / (float)i; // Calculate and add the term to the sum.
}
}
// Print the final result.
printf("\nSum of Series upto %d terms : %f \n", n, s);
}
Output:
Input the number of terms : 5 1/1 + 1/2 + 1/3 + 1/4 + 1/5 Sum of Series upto 5 terms : 2.283334
Flowchart:
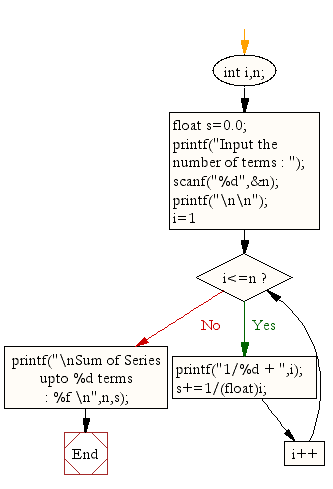
C Programming Code Editor:
Previous: Write a program in C to find the sum of the series [ 1-X^2/2!+X^4/4!- .........].
Next: Write a program in C to display the pattern like a pyramid using asterisk and each row contain an odd number of asterisks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics