C Exercises: Calculate the sum of the series [ 1-X^2/2!+X^4/4!- .........]
18. Sum of Series [1 - X²/2! + X⁴/4! - …]
Write a program in C to find the sum of the series [ 1-X^2/2!+X^4/4!- .........].
This C program calculates the sum of a series where the terms alternate between positive and negative, involving powers of X and factorial denominators: 1-X^2/2!+X^4/4!-.. . The program will take an input value for X and a specified number of terms, then compute the sum of the series using a loop to iterate through each term, applying the appropriate sign and factorial calculation.
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
float x, sum, t, d; // Declare variables to store input and intermediate values.
int i, n; // Declare variables for loop control and input.
// Prompt the user to input the value of 'x'.
printf("Input the Value of x :");
scanf("%f", &x); // Read the value of 'x' from the user.
// Prompt the user to input the number of terms.
printf("Input the number of terms : ");
scanf("%d", &n); // Read the value of 'n' from the user.
sum = 1; // Initialize 'sum' to 1, as the first term is always 1.
t = 1; // Initialize 't' to 1 for the first term.
// Loop to calculate the sum of the series.
for (i = 1; i < n; i++)
{
d = (2 * i) * (2 * i - 1); // Calculate the denominator for the term.
t = -t * x * x / d; // Calculate the term value.
sum = sum + t; // Add the term to the sum.
}
// Print the final result along with the input values.
printf("\nThe sum = %f\nNumber of terms = %d\nValue of x = %f\n", sum, n, x);
}
Flowchart:
or
#include <stdio.h>
void main()
{
float x,s,t,num=1.00,fac=1.00;
int i,n,pr,y=2,m=1;
printf("Input the Value of x :");
scanf("%f",&x);
printf("Input the number of terms : ");
scanf("%d",&n);
s=1.00; t=1.00;
for (i=1;i<n;i++)
{
for(pr=1;pr<=y;pr++)
{
fac=fac*pr;
num=num*x;
}
m=m*(-1);
num=num*m;
t=num/fac;
s=s+t;
y=y+2;
num=1.00;
fac=1.00;
}
printf("\nthe sum = %f\nNumber of terms = %d\nvalue of x = %f\n",s,n,x);
}
Output:
Input the Value of x :2 Input the number of terms : 5 the sum = -0.415873 Number of terms = 5 value of x = 2.000000
Flowchart:
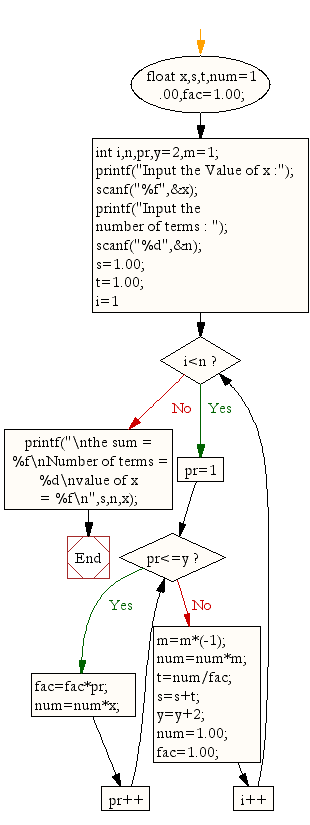
For more Practice: Solve these Related Problems:
- Write a C program to compute the alternating series for cosine of x using a do-while loop.
- Write a C program to calculate the series 1 - x²/2! + x⁴/4! - … using recursion for factorial computation.
- Write a C program to display each term of the series and then compute the overall sum.
- Write a C program to compute the series sum and compare the result with the cos(x) function from math.h.
C Programming Code Editor:
Previous: Write a program in C to make such a pattern like a pyramid with a number which will repeat the number in the same row.
Next: Write a program in C to display the n terms of harmonic series and their sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.