C Exercises: Display first 10 natural numbers
1. First 10 Natural Numbers Display
Write a program in C to display the first 10 natural numbers.
This C program demonstrates how to display the first 10 natural numbers. It uses a simple “for” loop to iterate from 1 to 10 and prints each number sequentially to the console. This basic program is an excellent introduction to loops and output in C.
Visual Presentation:
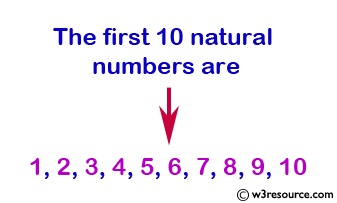
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int i; // Declare a variable 'i' for the loop counter.
printf("The first 10 natural numbers are:\n"); // Print a message to indicate the output.
for (i=1; i<=10; i++) // Start a for loop to iterate from 1 to 10.
{
printf("%d ", i); // Print the current value of 'i'.
}
printf("\n"); // Print a new line for better formatting.
}
Output:
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 9 10
Explanation:
for (i = 1; i <= 10; i++) { printf("%d ", i); }
In the above for loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to 10. In each iteration of the loop, the printf function will print the value of i to the console, followed by a space character.
Finally, the loop will increment the value of i by 1, and the process will repeat until the condition i<=10 is no longer true.
So, when this code runs, it will output the numbers 1 through 10 separated by spaces.
Flowchart:
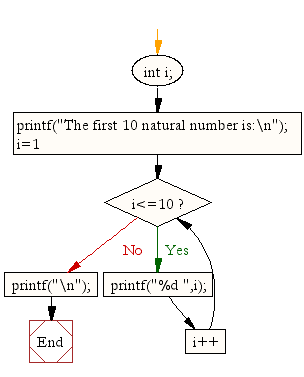
Step wise execution of C program :
- Green color means line that has just executed
- Red color means next line to excute
- Stack : a stack is an abstract data type that serves as a collection of elements.
Steps | Code | Stack | Output |
---|---|---|---|
Step-1 |
1 int main(){
2 int i;
3 printf("The first 10 natural numbers are:\n");
4 for (i=1;i<=10;i++)
5 {
6 printf("%d ",i);
7 }
8 printf("\n");
9 return 0;
10 }
|
main |
|
Step-2 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): ? |
|
Step-3 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): ? |
The first 10 natural numbers are: |
Step-4 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 1 |
The first 10 natural numbers are: |
Step-5 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 1 |
The first 10 natural numbers are: 1 |
Step-6 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 2 |
The first 5 natural numbers are: 1 |
Step-7 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 2 |
The first 5 natural numbers are: 1 2 |
Step-8 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 3 |
The first 5 natural numbers are: 1 2 |
Step-9 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 3 |
The first 5 natural numbers are: 1 2 3 |
Step-10 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 4 |
The first 10 natural numbers are: 1 2 3 |
Step-11 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 4 |
The first 10 natural numbers are: 1 2 3 4 |
Step-12 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 5 |
The first 10 natural numbers are: 1 2 3 4 |
Step-13 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 5 |
The first 10 natural numbers are: 1 2 3 4 5 |
Step-14 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 6 |
The first 10 natural numbers are: 1 2 3 4 5 |
Step-15 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 6 |
The first 10 natural numbers are: 1 2 3 4 5 6 |
Step-16 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 7 |
The first 10 natural numbers are: 1 2 3 4 5 6 |
Step-17 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 7 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 |
Step-18 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 8 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 |
Step-19 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 8 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 |
Step-20 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 9 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 |
Step-21 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 9 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 9 |
Step-22 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 10 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 9 |
Step-23 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 10 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 9 10 |
Step-24 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 11 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 9 10 |
Step-25 | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 11 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 9 10 |
Exit | 1 int main(){ 2 int i; 3 printf("The first 10 natural numbers are:\n"); 4 for (i=1;i<=10;i++) 5 { 6 printf("%d ",i); 7 } 8 printf("\n"); 9 return 0; 10 } |
main i(int): 11 |
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 9 10 |
For more Practice: Solve these Related Problems:
- Write a C program to display the first 10 natural numbers in reverse order using recursion.
- Write a C program to display the first 10 natural numbers using pointer arithmetic instead of array indexing.
- Write a C program to print the first 10 natural numbers without using any loop constructs (use recursion).
- Write a C program to shuffle the first 10 natural numbers randomly and then display them.
C Programming Code Editor:
Previous: C For Loop Exercises Home
Next: Write a C program to find the sum of first 10 natural numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.