C Exercises: Count the number of words and characters in a file
7. Count Words & Characters in File
Write a program in C to count the number of words and characters in a file.
To count the number of words and characters in a file using C, open the file for reading, then iterate through its contents character by character. Use a counter to keep track of characters and another to count words, incrementing the word counter each time you encounter a space or newline that follows a non-space character. Finally, output the counts of words and characters.
Pseudocode Plan
- File Opening:
- Open the file in read mode.
- Check if the file is successfully opened.
- Initialization:
- Initialize counters for words and characters.
- A flag to track if the last character read was a whitespace.
- File Reading Loop:
- Read characters from the file one by one.
- Print each character.
- Increment character counter if the character is not EOF.
- Check for word boundaries:
- Increment the word counter if the current character is not a whitespace and the last character was a whitespace.
- Update the last character read flag.
- Final Output:
- Print the total count of words and characters.
- Close File:
- Close the file.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
int main()
{
FILE *fptr;
char ch;
int words = 0, characters = 0;
int inWord = 0; // Flag to check if inside a word
char fname[20];
printf("\n\nCount the number of words and characters in a file:\n");
printf("---------------------------------------------------------\n");
printf("Input the filename to be opened: ");
scanf("%s", fname);
fptr = fopen(fname, "r");
if(fptr == NULL)
{
printf("File does not exist or cannot be opened.\n");
return 1;
}
printf("The content of the file %s are:\n", fname);
while ((ch = fgetc(fptr)) != EOF)
{
putchar(ch);
characters++;
if (isspace(ch))
{
inWord = 0;
}
else if (!inWord)
{
inWord = 1;
words++;
}
}
fclose(fptr);
printf("\nThe number of words in the file %s are: %d\n", fname, words);
printf("The number of characters in the file %s are: %d\n", fname, characters);
return 0;
}
Output:
Count the number of words and characters in a file: --------------------------------------------------------- Input the filename to be opened: test1.txt The content of the file test1.txt are: test line 1 test line 2 test line 3 test line 4 The number of words in the file test1.txt are: 12 The number of characters in the file test1.txt are: 50
Explanation:
- isspace(ch): This function checks if the character is a whitespace (space, newline, tab, etc.).
- inWord flag: Keeps track of whether we are inside a word or not.
- Word count: Incremented only when a non-whitespace character is found after a whitespace sequence.
- Character count: Incremented for every character read from the file.
Flowchart:
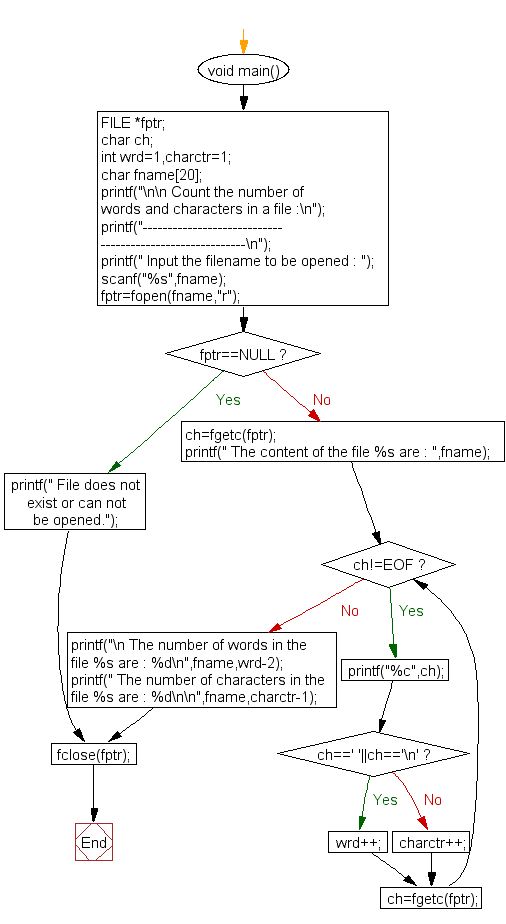
For more Practice: Solve these Related Problems:
- Write a C program to count the number of vowels and consonants in a file's content.
- Write a C program to compute and display the frequency of each letter from a text file.
- Write a C program to read a file and count the number of digits, whitespace, and punctuation characters.
- Write a C program to count the number of words in a file while ignoring numeric strings.
C Programming Code Editor:
Previous: Write a program in C to find the content of the file and number of lines in a Text File.
Next: Write a program in C to delete a specific line from a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.