C Exercises: Find the content of the file and number of lines in a Text File
Write a program in C to find the content of a file and the number of lines in a text file.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LSIZ 128
#define RSIZ 10
int main(void)
{
char line[RSIZ][LSIZ];
char fname[20];
FILE *fptr = NULL;
int i = 0;
int tot = 0;
printf("\n\n Find the content of the file and number of lines in a Text File :\n");
printf("----------------------------------------------------------------------\n");
printf(" Input the file name to be opened : ");
scanf("%s",fname);
fptr = fopen(fname, "r");
/*--------------------- store the lines into an array ----------------*/
while(fgets(line[i], LSIZ, fptr))
{
line[i][strlen(line[i]) - 1] = '\0';
i++;
}
tot = i;
printf("\n The content of the file %s are : \n",fname);
for(i = 0; i < tot; ++i)
{
printf(" %s\n", line[i]);
}
/*---------------------------------------------------------------------*/
printf("\n The lines in the file are : %d\n",tot-1);
printf("\n");
return 0;
}
Sample Output:
Find the content of the file and number of lines in a Text File : ---------------------------------------------------------------------- Input the file name to be opened : test.txt The content of the file test.txt are : test line 1 test line 2 test line 3 test line 4 The lines in the file are : 4
Flowchart:
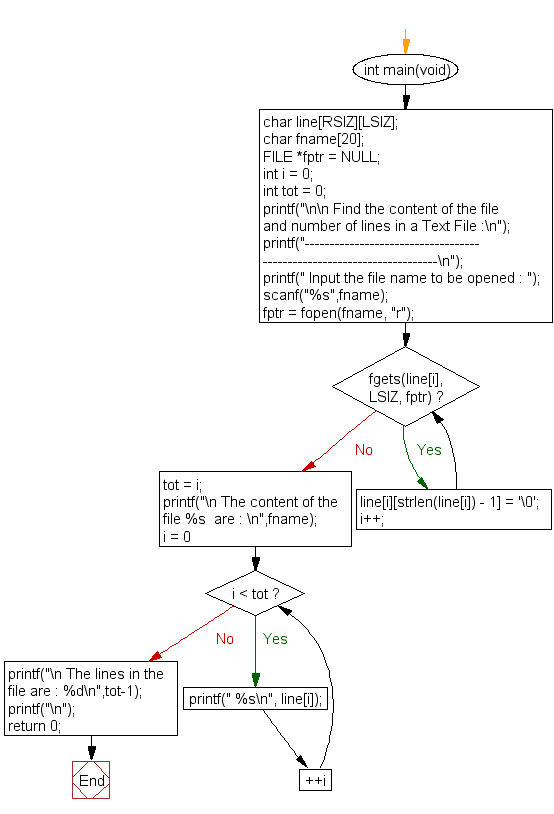
C Programming Code Editor:
Previous: Write a program in C to Find the Number of Lines in a Text File.
Next: Write a program in C to count number of words and characters in a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics