C Exercises: Read the file and store the lines into an array
4. Read File to Array
Write a program in C to read the file and store the lines in an array.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LSIZ 128
#define RSIZ 10
int main(void)
{
char line[RSIZ][LSIZ];
char fname[20];
FILE *fptr = NULL;
int i = 0;
int tot = 0;
printf("\n\n Read the file and store the lines into an array :\n");
printf("------------------------------------------------------\n");
printf(" Input the filename to be opened : ");
scanf("%s",fname);
fptr = fopen(fname, "r");
while(fgets(line[i], LSIZ, fptr))
{
line[i][strlen(line[i]) - 1] = '\0';
i++;
}
tot = i;
printf("\n The content of the file %s are : \n",fname);
for(i = 0; i < tot; ++i)
{
printf(" %s\n", line[i]);
}
printf("\n");
return 0;
}
Sample Output:
Read the file and store the lines into an array : ------------------------------------------------------ Input the filename to be opened : test.txt The content of the file test.txt are : test line 1 test line 2 test line 3 test line 4
Flowchart:
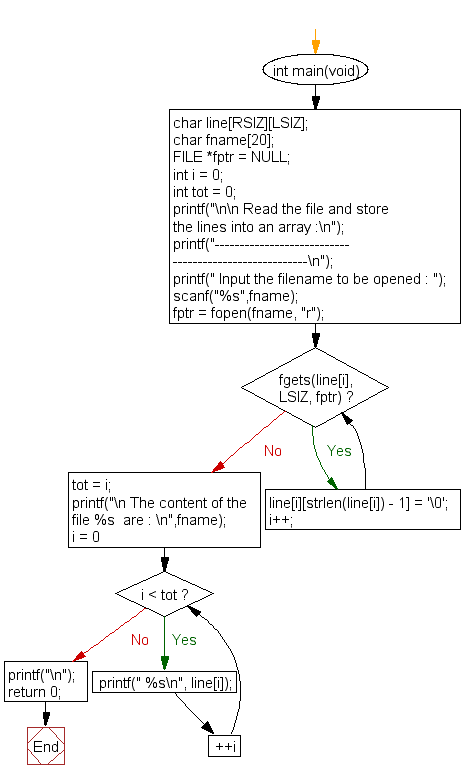
For more Practice: Solve these Related Problems:
- Write a C program to read a file line by line and store each line in a dynamically allocated array of strings.
- Write a C program to load file content into an array and then allow the user to search for a line by its index.
- Write a C program to read a file and reverse the order of lines stored in an array before printing them.
- Write a C program to read a file into an array, then sort the lines alphabetically and display the sorted output.
C Programming Code Editor:
Previous: Write a program in C to write multiple lines in a text file.
Next: Write a program in C to Find the Number of Lines in a Text File.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.