C Exercises: String representation of common directory paths
16. Common Directory Tree
A set of strings represent directory paths and a single character directory separator (/).
Write a program in C language to get a part of the directory tree that is common to all directories.
Test Data:
({/home/me/user/temp/a", "/home/me/user/temp/b","/home/me/user/temp/c/d"}) ->
/home/me/user/temp
Sample Solution:
C Code:
Sample Output:
Common path: /home/me/user/temp
Flowchart:
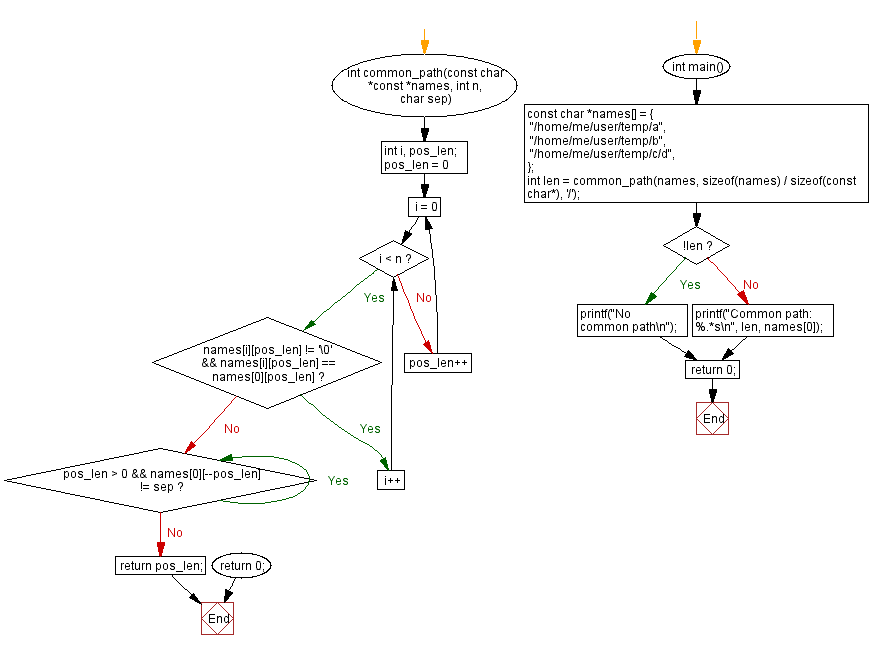
For more Practice: Solve these Related Problems:
- Write a C program to compare multiple directory paths and output the longest common prefix.
- Write a C program to extract the common subdirectory from a list of file paths with different separators.
- Write a C program to parse directory paths and display the directory tree structure that is shared by all paths.
- Write a C program to find the common root directory from a list of absolute paths and print it in normalized form.
C Programming Code Editor:
Previous: Remove a file from the disk.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics