C Exercises: Merge two files and write it in a new file
C File Handling : Exercise-12 with Solution
Write a program in C to merge two files and write them to another file.
Assume that the content of the file test.txt and test1.txr is : The content of the file test.txt is : This is the file test.txt. The content of the file test1.txt is : This is the file test1.txt.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
void main()
{
FILE *fold1, *fold2, *fnew;
char ch, fname1[20], fname2[20], fname3[30];
printf("\n\n Merge two files and write it in a new file :\n");
printf("-------------------------------------------------\n");
printf(" Input the 1st file name : ");
scanf("%s",fname1);
printf(" Input the 2nd file name : ");
scanf("%s",fname2);
printf(" Input the new file name where to merge the above two files : ");
scanf("%s",fname3);
fold1=fopen(fname1, "r");
fold2=fopen(fname2, "r");
if(fold1==NULL || fold2==NULL)
{
// perror("Error Message ");
printf(" File does not exist or error in opening...!!\n");
exit(EXIT_FAILURE);
}
fnew=fopen(fname3, "w");
if(fnew==NULL)
{
// perror("Error Message ");
printf(" File does not exist or error in opening...!!\n");
exit(EXIT_FAILURE);
}
while((ch=fgetc(fold1))!=EOF)
{
fputc(ch, fnew);
}
while((ch=fgetc(fold2))!=EOF)
{
fputc(ch, fnew);
}
printf(" The two files merged into %s file successfully..!!\n\n", fname3);
fclose(fold1);
fclose(fold2);
fclose(fnew);
}
Sample Output:
Merge two files and write it in a new file : ------------------------------------------------- Input the 1st file name : test.txt Input the 2nd file name : test1.txt Input the new file name where to merge the above two files : mergefiles.txt The two files merged into mergefiles.txt file successfully..!!
Flowchart:
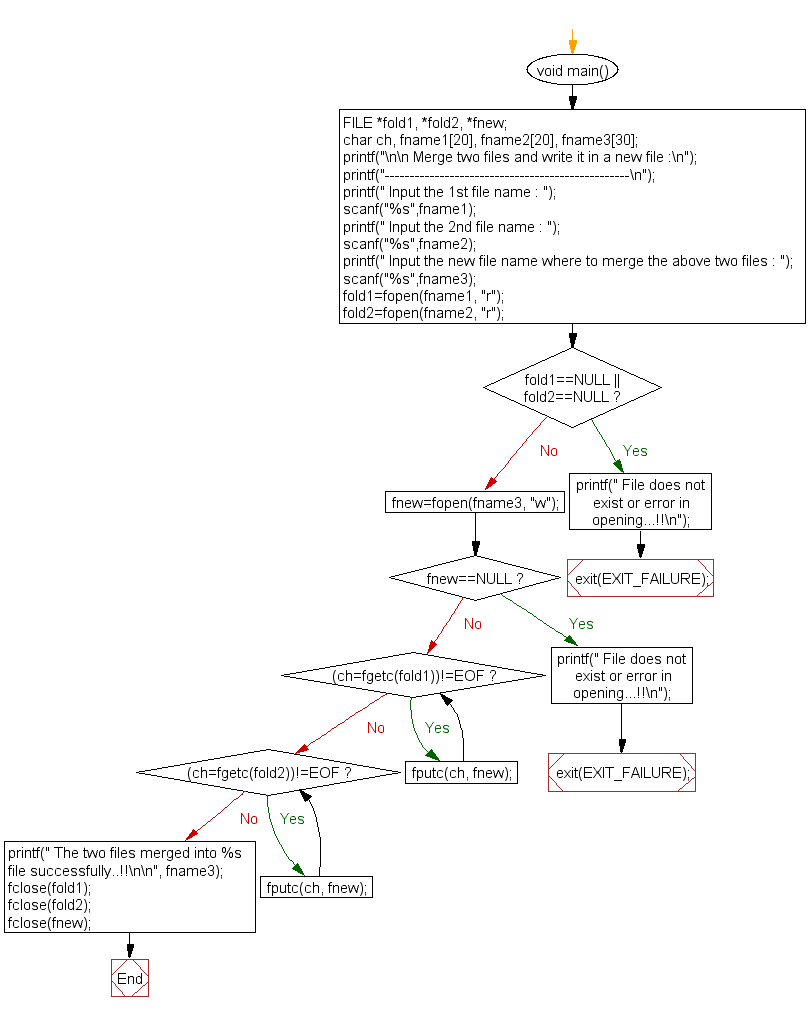
C Programming Code Editor:
Previous: Write a program in C to copy a file in another name.
Next: Write a program in C to encrypt a text file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics