C Exercises: Append multiple lines at the end of a text file
10. Append Multiple Lines to File
Write a program in C to append multiple lines to the end of a text file.
Assume that the content of the file test.txt is : test line 1 test line 2 test line 3 test line 4
Sample Solution:
C Code:
#include <stdio.h>
int main ()
{
FILE * fptr;
int i,n;
char str[100];
char fname[20];
char str1;
printf("\n\n Append multiple lines at the end of a text file :\n");
printf("------------------------------------------------------\n");
printf(" Input the file name to be opened : ");
scanf("%s",fname);
fptr = fopen(fname, "a");
printf(" Input the number of lines to be written : ");
scanf("%d", &n);
printf(" The lines are : \n");
for(i = 0; i < n+1;i++)
{
fgets(str, sizeof str, stdin);
fputs(str, fptr);
}
fclose (fptr);
//----- Read the file after appended -------
fptr = fopen (fname, "r");
printf("\n The content of the file %s is :\n",fname);
str1 = fgetc(fptr);
while (str1 != EOF)
{
printf ("%c", str1);
str1 = fgetc(fptr);
}
printf("\n\n");
fclose (fptr);
//------- End of reading ------------------
return 0;
}
Sample Output:
Append multiple lines at the end of a text file : ------------------------------------------------------ Input the file name to be opened : test.txt Input the number of lines to be written : 3 The lines are : test line 5 test line 6 test line 7 The content of the file test.txt is : test line 1 test line 2 test line 3 test line 4 test line 5 test line 6 test line 7
Flowchart:
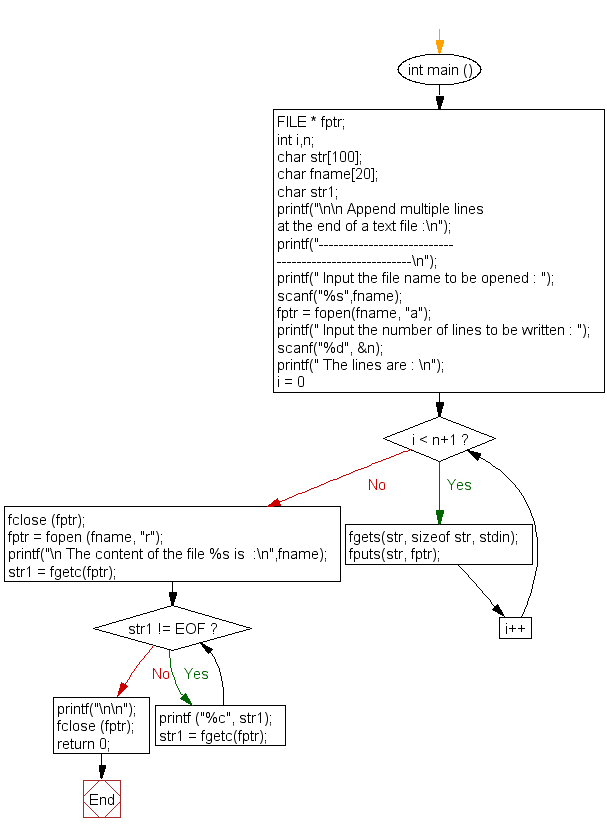
For more Practice: Solve these Related Problems:
- Write a C program to append a block of text with a header and footer to the end of an existing file.
- Write a C program to append user-input lines to a file and then display only the newly added lines.
- Write a C program to append lines to a file only if they do not already exist in the file.
- Write a C program to append multiple lines to a file and timestamp each appended line.
C Programming Code Editor:
Previous: Write a program in C to replace a specific line with another text in a file.
Next: Write a program in C to copy a file in another name.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics