C Exercises: Create a file and input text
1. Create & Store File
Write a program in C to create and store information in a text file.
Sample Solution:
#include <stdio.h>
#include <stdlib.h>
int main()
{
char str[1000];
FILE *fptr;
char fname[20]="test.txt";
printf("\n\n Create a file (test.txt) and input text :\n");
printf("----------------------------------------------\n");
fptr=fopen(fname,"w");
if(fptr==NULL)
{
printf(" Error in opening file!");
exit(1);
}
printf(" Input a sentence for the file : ");
fgets(str, sizeof str, stdin);
fprintf(fptr,"%s",str);
fclose(fptr);
printf("\n The file %s created successfully...!!\n\n",fname);
return 0;
}
Sample Output:
Create a file (test.txt) and input text : ---------------------------------------------- Input a sentence for the file : This is the content of the file test.txt The file test.txt created successfully...!!
Flowchart:
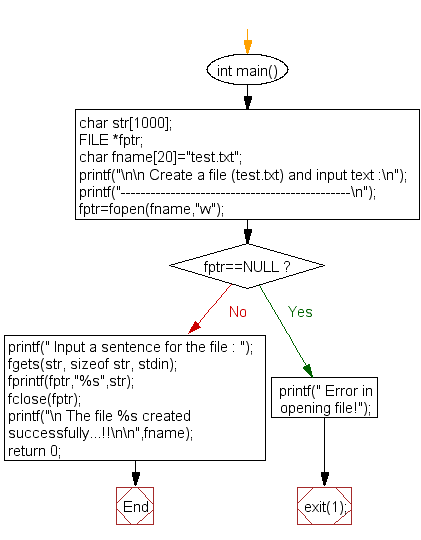
For more Practice: Solve these Related Problems:
- Write a C program to create a text file and write user input to it line by line until a termination string is entered.
- Write a C program to create a text file with a timestamp in its name and store a fixed paragraph into it.
- Write a C program to create a file and then append system environment information to it using file I/O functions.
- Write a C program to create a file and then write both numeric and string data formatted in a table.
C Programming Code Editor:
Previous: C File Handling Exercises Home
Next: Write a program in C to read an existing file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.