C Program: Calculate Sum of squares of digits with Do-While Loop
6. Sum of Squares of Digits
Write a C program that prompts the user to enter a positive integer and then calculates and prints the sum of the squares of each digit in that number using a do-while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int num, digit, sum = 0;
// Prompt the user to enter a positive integer
printf("Input a positive integer: ");
scanf("%d", &num);
// Check if the entered number is positive
if (num <= 0) {
printf("Please input a positive integer.\n");
return 1; // Return an error code
}
// Calculate the sum of the squares of each digit
do {
digit = num % 10; // Extract the last digit
sum += digit * digit; // Add the square of the digit to the sum
num /= 10; // Remove the last digit
} while (num != 0); // Continue the loop until there are no more digits
// Print the sum of the squares of each digit
printf("Sum of the squares of each digit: %d\n", sum);
return 0; // Indicate successful program execution
}
Sample Output:
Input a positive integer: 1234 Sum of the squares of each digit: 30
Explanation:
Here are key parts of the above code step by step:
- int num, digit, sum = 0;: Declares variables to store the entered number ('num'), each digit ('digit'), and the sum of the squares of digits ('sum').
- printf("Input a positive integer: ");: Prompts the user to input a positive integer.
- scanf("%d", &num);: Reads the entered integer from the user and stores it in the variable num.
- if (num <= 0) { ... }: Checks if the entered number is positive. If not, it displays an error message and returns an error code.
- do { ... } while (num != 0);: Starts a do-while loop to iterate through each digit of the entered number.
- digit = num % 10;: Extracts the last digit of the number.
- sum += digit * digit;: Adds the square of the extracted digit to the sum.
- num /= 10;: Removes the last digit from the number.
- The loop continues until there are no more digits in the number.
- printf("Sum of the squares of each digit: %d\n", sum);: Prints the calculated sum of the squares of each digit.
- return 0;: Indicates successful program execution.
Flowchart:
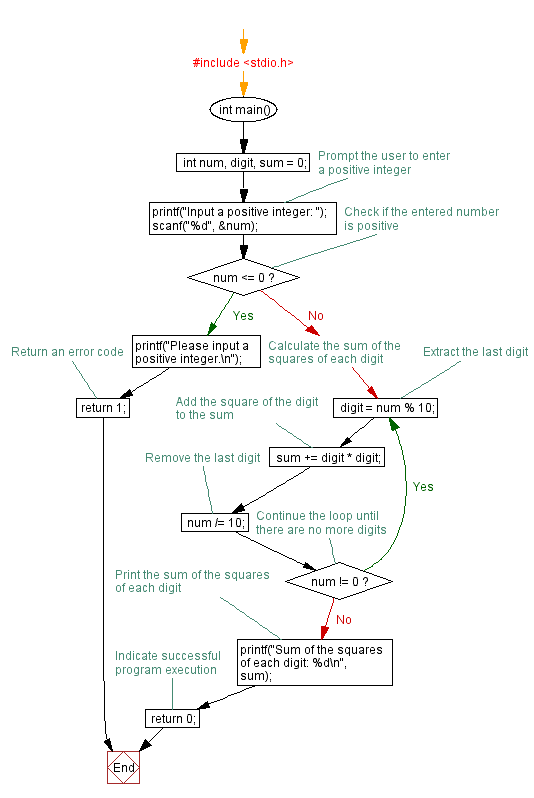
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of cubes of digits of a given number using a do-while loop.
- Write a C program to find the sum of squares of digits of a given number, but exclude squares of odd digits.
- Write a C program to find the sum of squares of digits and check if the sum is a prime number.
- Write a C program to compute the sum of squares of digits until the sum itself is a single-digit number.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Guess the number game with Do-While Loop.
Next: Implement a stack using a singly linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.