C Program: Calculate Sum of entered numbers with Do-While Loop
4. Sum Until Negative Number
Write a C program that prompts the user to enter a series of numbers until they input a negative number. Calculate and print the sum of all entered numbers using a do-while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int num, sum = 0; // Declare variables to store the entered number and the sum
// Prompt the user to enter numbers until they input a negative number
do {
printf("Input a number (input a negative number to stop): ");
scanf("%d", &num); // Read the entered number
if (num >= 0) {
sum += num; // Add the number to the sum if it's non-negative
}
} while (num >= 0); // Continue the loop until the user enters a negative number
// Print the sum of all entered numbers
printf("Sum of entered numbers: %d\n", sum);
return 0; // Indicate successful program execution
}
Sample Output:
Input a number (input a negative number to stop): 2 Input a number (input a negative number to stop): 3 Input a number (input a negative number to stop): 0 Input a number (input a negative number to stop): 1 Input a number (input a negative number to stop): 56 Input a number (input a negative number to stop): 1 Input a number (input a negative number to stop): -12 Sum of entered numbers: 63
Explanation:
Here is the break down of the above code step by step:
- int num, sum = 0;: Declares two integer variables, 'num' to store the entered number, and 'sum' to store the sum of entered numbers. 'sum' is initialized to 0.
- do {: Marks the beginning of a do-while loop. The code inside the loop will be executed at least once before checking the loop condition.
- printf("Input a number (input a negative number to stop): ");: Displays a prompt asking the user to enter a number, with an additional instruction to enter a negative number to stop.
- scanf("%d", &num);: Reads the entered number from the user and stores it in the variable 'num'.
- if (num >= 0) {: Checks if the entered number is non-negative.
- sum += num;: If the number is non-negative, it is added to the 'sum'.
- } while (num >= 0);: Specifies the loop condition. The loop continues as long as the user enters a non-negative number.
- printf("Sum of entered numbers: %d\n", sum);: Prints the sum of all entered numbers.
- return 0;: Indicates successful program execution.
- }: Marks the end of the "main()" function.
Flowchart:
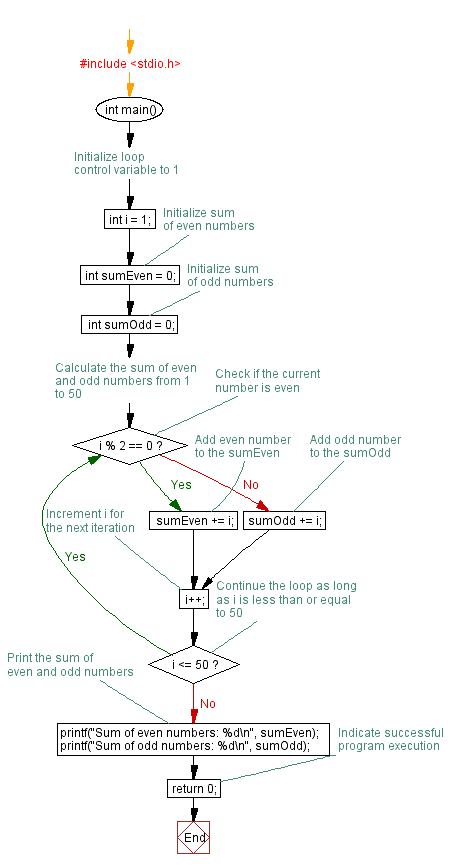
For more Practice: Solve these Related Problems:
- Write a C program to accept numbers until a negative number is entered and print the product of all positive numbers.
- Write a C program to accept numbers until a negative number is entered and find the maximum number entered.
- Write a C program to accept numbers until a negative number is entered and display the average of all entered numbers.
- Write a C program to accept numbers until a negative number is entered and print the sum of only odd numbers.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate Sum of even and odd numbers (1-50) with Do-While Loops.
Next: C Program: Guess the number game with Do-While Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.