C Program: Calculate Sum of even and odd numbers (1-50) with Do-While Loops
3. Sum of Even and Odd Numbers
Write a C program that calculates the sum of even and odd numbers from 1 to 50 using do-while loops.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int i = 1; // Initialize loop control variable to 1
int sumEven = 0; // Initialize sum of even numbers
int sumOdd = 0; // Initialize sum of odd numbers
// Calculate the sum of even and odd numbers from 1 to 50
do {
// Check if the current number is even
if (i % 2 == 0) {
sumEven += i; // Add even number to the sumEven
} else {
sumOdd += i; // Add odd number to the sumOdd
}
i++; // Increment i for the next iteration
} while (i <= 50); // Continue the loop as long as i is less than or equal to 50
// Print the sum of even and odd numbers
printf("Sum of even numbers: %d\n", sumEven);
printf("Sum of odd numbers: %d\n", sumOdd);
return 0; // Indicate successful program execution
}
Sample Output:
Sum of even numbers: 650 Sum of odd numbers: 625
Explanation:
Here is the break down of the above code step by step:
- int i = 1;: Initializes the loop control variable i to 1.
- int sumEven = 0;: Initializes the variable 'sumEven' to store the sum of even numbers.
- int sumOdd = 0;: Initializes the variable 'sumOdd' to store the sum of odd numbers.
- do {: Marks the beginning of the do-while loop. The code inside the loop will be executed at least once before checking the loop condition.
- if (i % 2 == 0) {: Checks if the current value of 'i' is even.
- sumEven += i;: If the current number is even, it is added to the 'sumEven'.
- } else {: If the current number is not even (i.e., odd), this block is executed.
- sumOdd += i;: Adds the odd number to the 'sumOdd'.
- i++;: Increments the loop control variable for the next iteration.
- } while (i <= 50);: Specifies the loop condition. The loop continues as long as 'i' is less than or equal to 50.
- printf("Sum of even numbers: %d\n", sumEven);: Prints the sum of even numbers.
- printf("Sum of odd numbers: %d\n", sumOdd);: Prints the sum of odd numbers.
- return 0;: Indicates successful program execution.
- }: Marks the end of the "main()" function.
Flowchart:
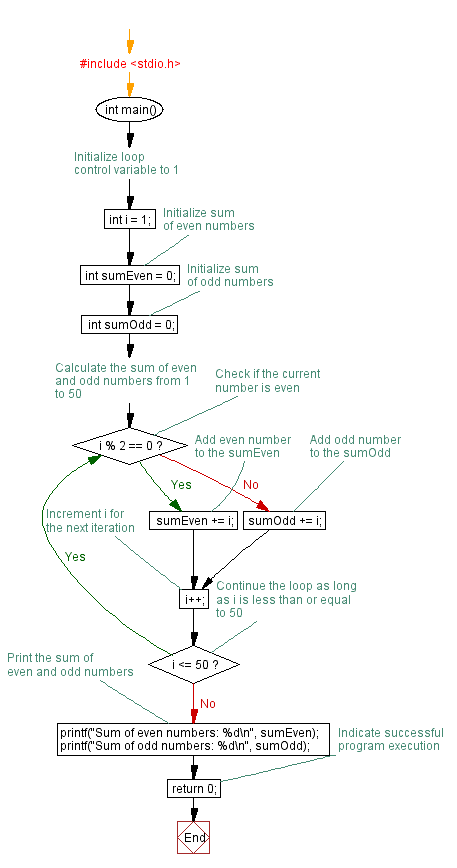
For more Practice: Solve these Related Problems:
- Write a C program to calculate the sum of only prime numbers from 1 to 50 using a do-while loop.
- Write a C program to find the sum of all numbers from 1 to 50 that are divisible by both 3 and 5.
- Write a C program to calculate the sum of even numbers from 1 to 50 but exclude numbers divisible by 4.
- Write a C program to compute the sum of all odd numbers up to 50 while skipping every third odd number.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate Sum of positive integers using Do-While Loop.
Next: Calculate Sum of entered numbers with Do-While Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.