C Exercises: Convert a tm object to custom textual representation
C Date Time: Exercise-4 with Solution
Write a program in C to convert a tm object to a custom textual representation.
Sample Solution:
C Code:
#include <stdio.h>
#include <time.h>
#include <locale.h>
int main(void) {
char time_buff[70]; // Buffer to store the formatted time string
// Create a custom time structure with specified date and time
struct tm mytime = {
.tm_year = 116, // = year 2016 (years since 1900)
.tm_mon = 8, // = 9th month (0-based index)
.tm_mday = 2, // = 2nd day of the month
.tm_hour = 16, // = 16 hours
.tm_min = 30, // = 30 minutes
.tm_sec = 32 // = 32 seconds
};
printf("\n The textual representation of specified date and time :\n");
// Convert the time structure to a formatted string according to the locale settings
if (strftime(time_buff, sizeof time_buff, "%B %c %P", &mytime)) {
puts(time_buff); // Print the formatted time string
} else {
puts("strftime failed"); // Print a message if strftime fails
}
// Change the locale to Greek (el_GR.utf8)
setlocale(LC_TIME, "el_GR.utf8");
// Format the time again after changing the locale
if (strftime(time_buff, sizeof time_buff, "%B %c %P", &mytime)) {
puts(time_buff); // Print the formatted time string in the Greek locale
} else {
puts("strftime failed"); // Print a message if strftime fails after changing the locale
}
printf("\n");
return 0;
}
Sample Output:
The textual representation of specified date and time : September Sun Sep 2 16:30:32 2016 pm September Sun Sep 2 16:30:32 2016 pm
Flowchart:
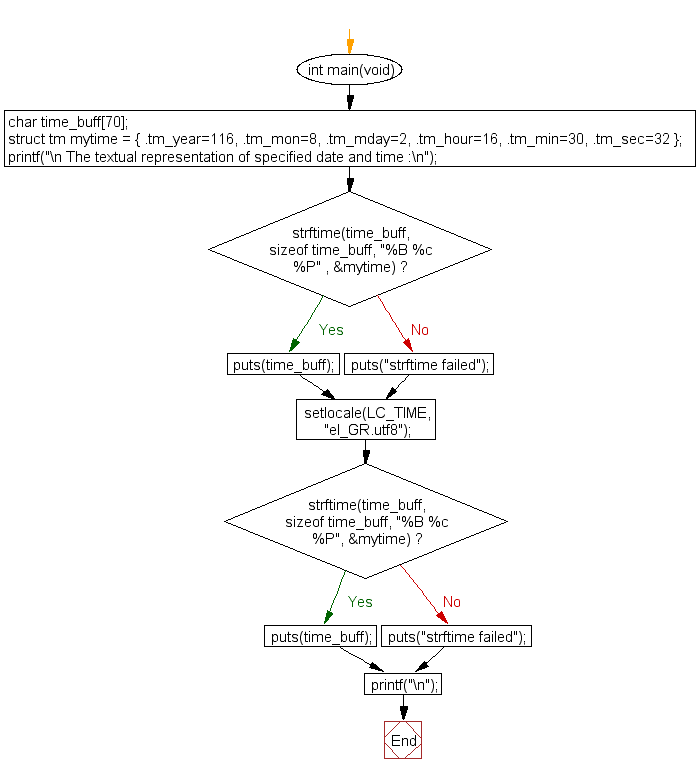
C Programming Code Editor:
Previous: Write a program in C to convert a time_t object to a textual representation.
Next: Write a program in C to convert a tm object to custom wide string textual representation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics