C Exercises: Compute the number of seconds passed since the beginning of the month
C Date Time: Exercise-2 with Solution
Write a program in C to compute the number of seconds passed since the beginning of the month.
Sample Solution:
C Code:
#include <stdio.h>
#include <time.h>
int main(void) {
time_t now; // Variable to hold the current time
time(&now); // Get the current time
struct tm beg_month; // Structure to hold the beginning of the month's date and time
beg_month = *localtime(&now); // Retrieve local time
beg_month.tm_hour = 0; // Set hours to 0
beg_month.tm_min = 0; // Set minutes to 0
beg_month.tm_sec = 0; // Set seconds to 0
beg_month.tm_mday = 1; // Set day to 1 (beginning of the month)
double seconds = difftime(now, mktime(&beg_month)); // Calculate the difference between the current time and the beginning of the month
printf("\n %.f seconds passed since the beginning of the month.\n\n", seconds); // Print the seconds elapsed since the beginning of the month
return 0;
}
Sample Output:
222084 seconds passed since the beginning of the month.
N.B.: The result may varry for your current system date and time.
Flowchart:
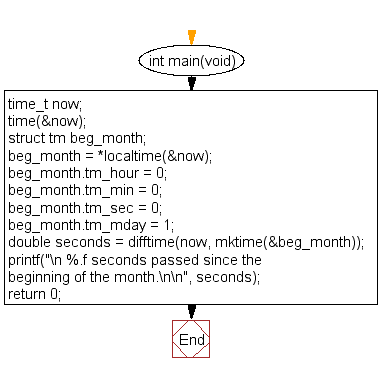
C Programming Code Editor:
Previous: Write a program in C to print the current date and time.
Next: Write a program in C to convert a time_t object to a textual representation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics