C Exercises: A menu-driven program for a simple calculator
C Conditional Statement: Exercise-26 with Solution
Write a program in C which is a Menu-Driven Program to perform a simple calculation.
Visual Presentation:
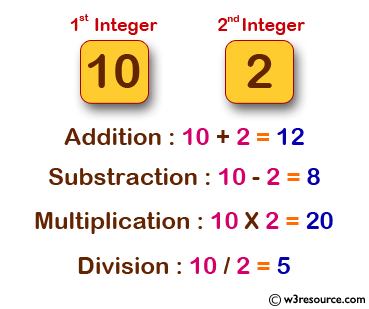
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int num1,num2,opt; // Declare variables to store user input and operation choice.
// Prompt user for two integers and store them.
printf("Enter the first Integer :");
scanf("%d",&num1);
printf("Enter the second Integer :");
scanf("%d",&num2);
// Display the menu for operation choice.
printf("\nInput your option :\n");
printf("1-Addition.\n2-Substraction.\n3-Multiplication.\n4-Division.\n5-Exit.\n");
scanf("%d",&opt); // Read and store the user's choice.
switch(opt) { // Start a switch statement based on the user's choice.
case 1:
printf("The Addition of %d and %d is: %d\n",num1,num2,num1+num2); // Perform addition and print result.
break;
case 2:
printf("The Substraction of %d and %d is: %d\n",num1,num2,num1-num2); // Perform subtraction and print result.
break;
case 3:
printf("The Multiplication of %d and %d is: %d\n",num1,num2,num1*num2); // Perform multiplication and print result.
break;
case 4:
if(num2==0) {
printf("The second integer is zero. Divide by zero.\n"); // Handle division by zero.
} else {
printf("The Division of %d and %d is : %d\n",num1,num2,num1/num2); // Perform division and print result.
}
break;
case 5:
break; // Exit the program.
default:
printf("Input correct option\n"); // Display error message for invalid input.
break;
}
}
Sample Output:
Enter the first Integer :10 Enter the second Integer :2 Input your option : 1-Addition. 2-Substraction. 3-Multiplication. 4-Division. 5-Exit. 3 The Multiplication of 10 and 2 is: 20
Flowchart:
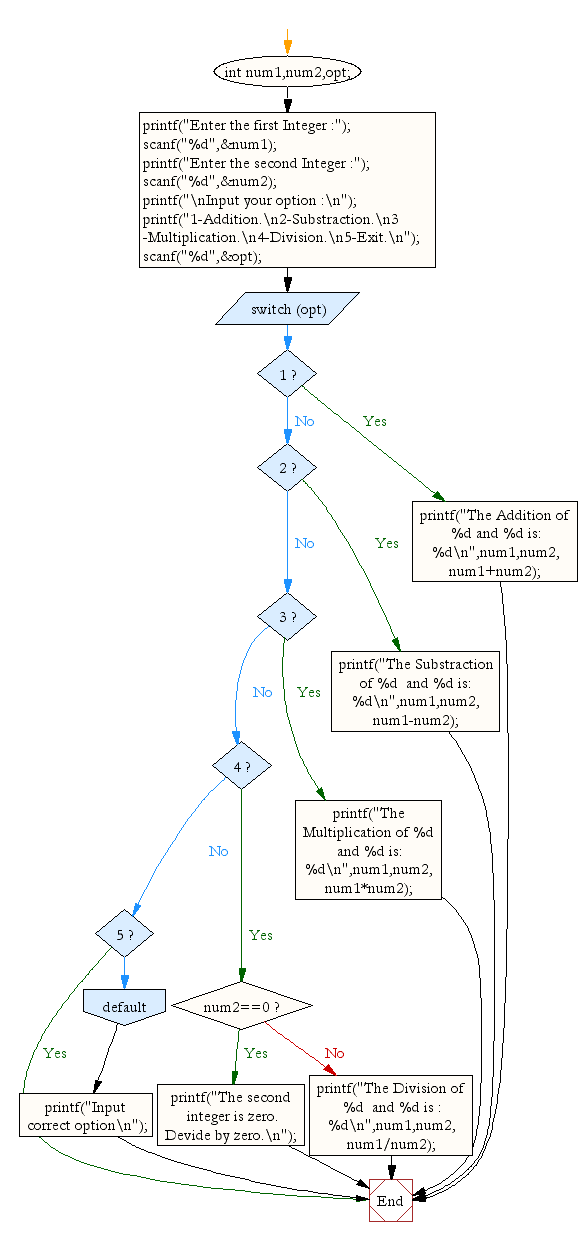
C Programming Code Editor:
Previous: Write a program in C which is a Menu-Driven Program to compute the area of the various geometrical shape.
Next: C For Loop Exercises Home
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics