C Exercises: Accept digit and display in the word
C Conditional Statement: Exercise-22 with Solution
Write a program in C to read any digit and display it in the word.
Visual Presentation:
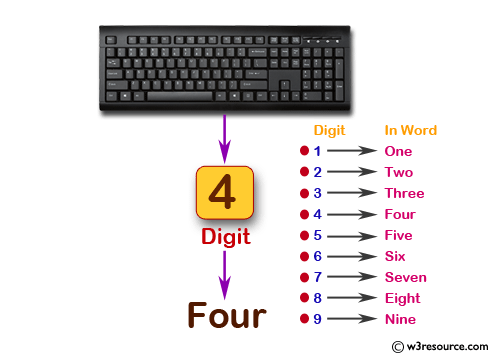
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int cdigit; // Declare a variable to store the input digit.
printf("Input Digit(0-9) : "); // Prompt the user to input a digit.
scanf("%d",&cdigit); // Read and store the input digit.
switch(cdigit) // Start a switch statement based on the input digit.
{
case 0:
printf("Zero\n"); // Print "Zero" for input digit 0.
break;
case 1:
printf("One\n"); // Print "One" for input digit 1.
break;
case 2:
printf("Two\n"); // Print "Two" for input digit 2.
break;
case 3:
printf("Three\n"); // Print "Three" for input digit 3.
break;
case 4:
printf("Four\n"); // Print "Four" for input digit 4.
break;
case 5:
printf("Five\n"); // Print "Five" for input digit 5.
break;
case 6:
printf("Six\n"); // Print "Six" for input digit 6.
break;
case 7:
printf("Seven\n"); // Print "Seven" for input digit 7.
break;
case 8:
printf("Eight\n"); // Print "Eight" for input digit 8.
break;
case 9:
printf("Nine\n"); // Print "Nine" for input digit 9.
break;
default:
printf("Invalid digit. \nPlease try again ....\n"); // Print a message for an invalid input.
break;
}
}
Sample Output:
Input Digit(0-9) : 4 Four
Flowchart:
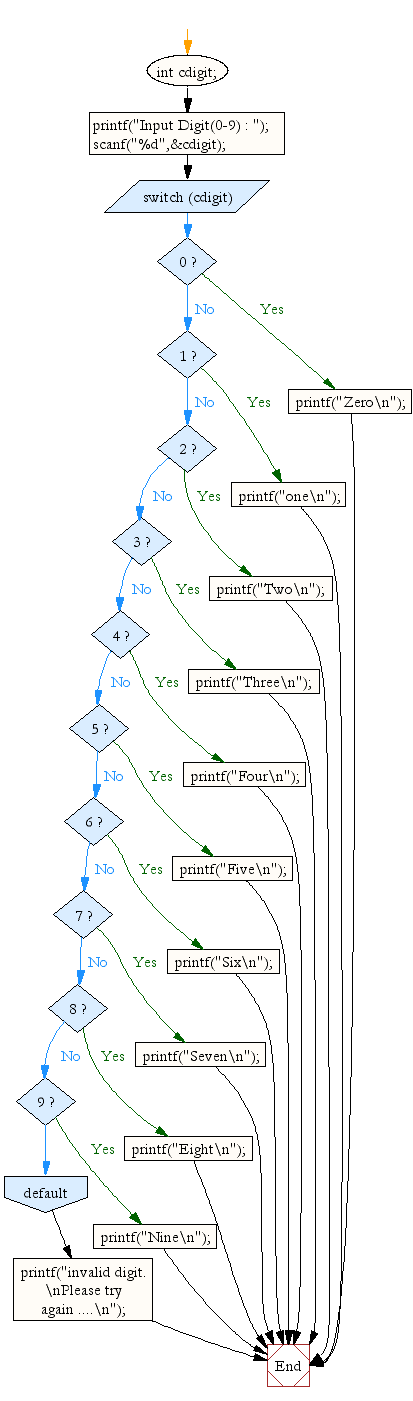
C Programming Code Editor:
Previous: Write a program in C to read any day number in integer and display day name in the word.
Next: Write a program in C to read any Month Number in integer and display Month name in the word.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/conditional-statement/c-conditional-statement-exercises-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics