C Exercises: Check whether two integers are equal or not
C Conditional Statement: Exercise-1 with Solution
Write a C program to accept two integers and check whether they are equal or not.
Visual Presentation:
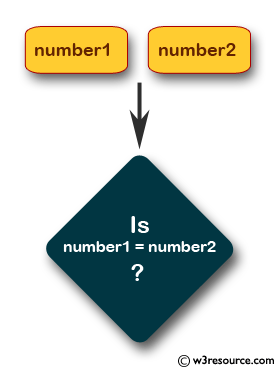
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int int1, int2; // Declare two integer variables 'int1' and 'int2'.
printf("Input the values for Number1 and Number2 : "); // Prompt the user to input values for Number1 and Number2.
scanf("%d %d", &int1, &int2); // Read and store the user's input in 'int1' and 'int2'.
if (int1 == int2) // Check if Number1 is equal to Number2.
printf("Number1 and Number2 are equal\n"); // Print a message if Number1 and Number2 are equal.
else
printf("Number1 and Number2 are not equal\n"); // Print a message if Number1 and Number2 are not equal.
}
Sample Output:
Input the values for Number1 and Number2 : 15 15 Number1 and Number2 are equal
Flowchart:
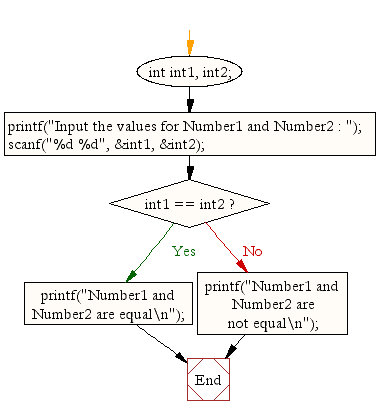
C Programming Code Editor:
Previous: C Conditional Statement Exercises Home
Next: Write a C program to check whether a given number is even or odd.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/conditional-statement/c-conditional-statement-exercises-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics