C Exercises: Remove all whitespace from a string
7. Whitespace Removal Callback Variants
Write a C program to remove all whitespace from a string using a callback function.
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
#include <ctype.h>
void remove_whitespace(char * str, void( * modify)(char * )) {
int i, j = 0;
for (i = 0; str[i] != '\0'; i++) {
if (!isspace(str[i])) {
str[j] = str[i];
j++;
} else {
modify( & str[i]);
}
}
str[j] = '\0';
}
void remove_space(char * ch) {
* ch = '\0';
}
int main() {
char str[100];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
str[strcspn(str, "\n")] = '\0'; // remove the newline character
printf("Original string: %s\n", str);
// remove whitespace using callback function
remove_whitespace(str, remove_space);
printf("String without whitespace: %s\n", str);
return 0;
}
Sample Output:
Enter a string: example . com Original string: example . com String without whitespace: example.com
Explanation:
In the above example 'remove_whitespace' function takes a string str and a function pointer modify as arguments. It loops through each character of the string using a for loop. If the current character is not a whitespace character (determined using the isspace function from the ctype.h library), it copies the character to the output string at position j and increments j.
If the current character is a whitespace character, it calls the modify function with a pointer to the current character.
The 'remove_space' function is the callback function that modifies each whitespace character. It simply replaces the whitespace character with a null character ('\0'), effectively removing it from the string.
The main() function reads a string from the user using fgets() function and removes the newline character at the end of the string using strcspn and assignment of '\0'. It then calls remove_whitespace with the input string and the remove_space callback function. Finally printf() function prints the modified string without whitespace.
Flowchart:
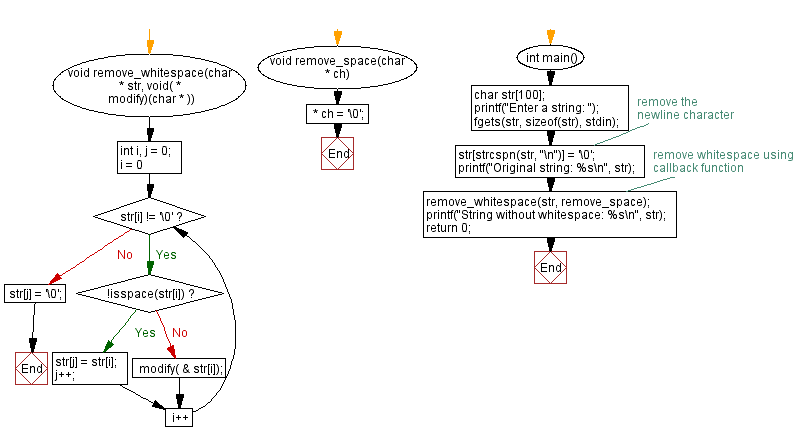
For more Practice: Solve these Related Problems:
- Write a C program to remove all spaces and punctuation from a string using a callback function.
- Write a C program to trim leading and trailing whitespace from a string using a callback.
- Write a C program to reduce multiple consecutive spaces in a string to a single space using a callback function.
- Write a C program to remove newline and tab characters from a string using a callback function.
C Programming Code Editor:
Previous: Calculate the average or median.
Next: Largest or smallest element in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.