C Exercises: Accepts some text from the user and prints each word of that text in separate line.
Print each word of input text on a new line
Write a C program that accepts some text from the user and prints each word of that text on a separate line.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
long nc = 0; // Variable to count characters
int new_l = 0; // Variable to count newlines
int n_word = 0; // Variable to count words
int chr; // Variable to hold input characters
int flag = 0; // Flag to track word boundaries
int last = 0; // Flag to track the last character
printf("Input some text:\n");
while ((chr = getchar()) != EOF) {
++nc; // Increment the count of characters
if (chr == ' ' || chr == '\t') {
flag = 0; // Reset the flag when a space or tab is encountered
} else if (chr == '\n') {
++new_l; // Increment the count of newlines
flag = 0; // Reset the flag on a newline
} else {
if (flag == 0) {
++n_word; // Increment the count of words when a new word begins
}
flag = 1; // Set the flag to indicate a word is in progress
}
if (flag == 0 && last == 0) {
printf("\n"); // Print a newline when a word ends and the last character was not a space
last = 1; // Set last to 1 to indicate the last character was a space
} else {
putchar(chr); // Print the character
last = 0; // Set last to 0 to indicate the last character was not a space
}
}
}
Sample Output:
Input some text: The quick brown fox jumps over the lazy dog The quick brown fox jumps over the lazy dog
Flowchart:
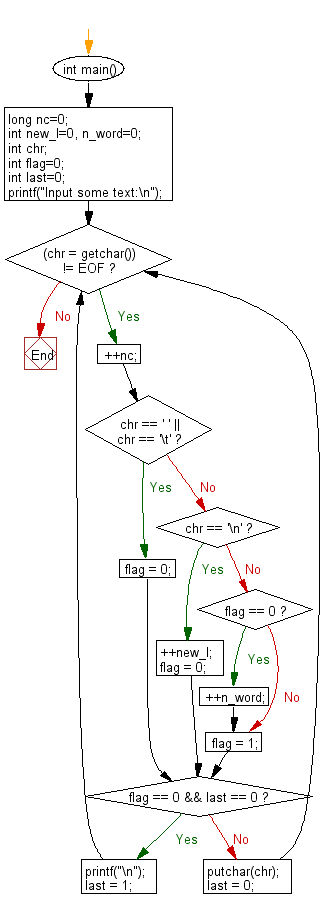
For more Practice: Solve these Related Problems:
- Write a C program to split an input sentence into words and print each word on a separate line without using strtok.
- Write a C program to print each word of a user-input string on a new line using pointer manipulation.
- Write a C program to break a sentence into words and output them line-by-line with proper handling of punctuation.
- Write a C program to process a string and display each word on a new line using a loop and character comparisons.
C programming Code Editor:
Previous:Write a C program to replace more than one blanks with a single blank in a input string.
Next: Write a C program that takes some integer values from the user and print a histogram.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.