C Exercises: Count blanks, tabs, and newlines in an input text.
Count blanks, tabs, and newlines in a text input
Write a C program to count blanks, tabs, and newlines in input text.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int blank_char, tab_char, new_line; // Variables to count blank characters, tab characters, and newlines
blank_char = 0; // Initialize the count of blank characters
tab_char = 0; // Initialize the count of tab characters
new_line = 0; // Initialize the count of newlines
int c; // Variable to hold input characters
printf("Number of blanks, tabs, and newlines:\n");
printf("Input few words/tab/newlines\n");
// Loop to read characters until end-of-file (EOF) is encountered
for (; (c = getchar()) != EOF;) {
if (c == ' ') {
++blank_char; // Increment the count of blank characters
}
if (c == '\t') {
++tab_char; // Increment the count of tab characters
}
if (c == '\n') {
++new_line; // Increment the count of newlines
}
}
// Print the final counts of blanks, tabs, and newlines
printf("blank=%d,tab=%d,newline=%d\n", blank_char, tab_char, new_line);
}
Sample Output:
Number of blanks, tabs, and newlines: Input few words/tab/newlines The quick brown fox jumps over the lazy dog ^Z blank=7,tab=2,newline=3
Flowchart:
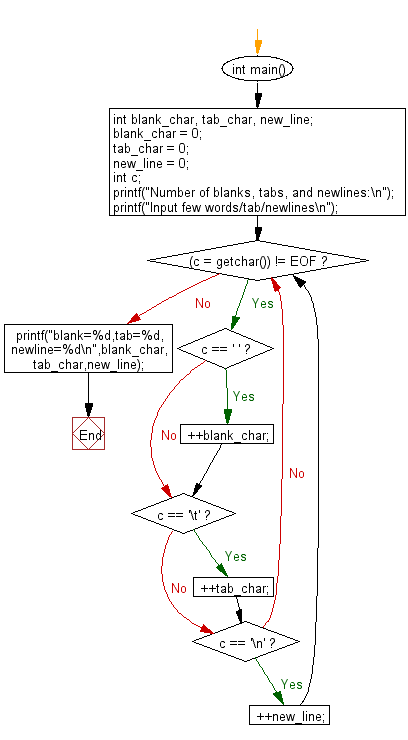
For more Practice: Solve these Related Problems:
- Write a C program to count the number of blanks, tabs, and newlines from text input until EOF.
- Write a C program to count and display the frequency of each whitespace character in user-provided text.
- Write a C program to compute the counts of spaces, tabs, and newline characters in multi-line input using a loop.
- Write a C program to read text from standard input and output clear counts for spaces, tabs, and newlines.
C programming Code Editor:
Previous:Write a C program to print the corresponding Fahrenheit to Celsius and Celsius to Fahrenheit.
Next: Write a C program to replace more than one blanks with a single blank in a input string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.