C Exercises: Calculate for each pair of integers x, y and z
Compute (x+y+z) for integers in valid range
Write a C program to calculate (x + y + z) for each pair of integers x, y and z where -2^31 <= x, y, z<= 2^31-1 .
Sample Solution:
C Code:
#include <stdio.h>
int main()
{
// Declare variables
long long x, y, z;
// Prompt the user for input
printf("Input x, y, z:\n");
// Read input values
scanf("%lld %lld %lld", &x, &y, &z);
// Calculate and print the result
printf("Result: %lld\n", x + y + z);
return 0; // Indicate successful execution of the program
}
Sample Output:
Input x,y,x: Result: 140733606875472
Flowchart:
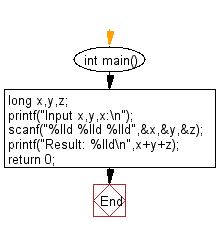
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of three integers ensuring the result remains within 32-bit limits.
- Write a C program to safely compute (x+y+z) and check for integer overflow before displaying the result.
- Write a C program to calculate the sum of three integers using the long long data type to prevent overflow.
- Write a C program to compute (x+y+z) with input validation ensuring each integer falls within the specified range.
C programming Code Editor:
Previous:Write a C program to create an extended ASCII table. Print the ASCII values 32 through 255.
Next: Write a C program to find all prime palindromes in the range of two given numbers x and y.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.