C Exercises: Reads the side of a square and prints square using hash (#) character
C Basic Declarations and Expressions: Exercise-80 with Solution
Write a C program that reads the side (side sizes between 1 and 10 ) of a square and prints square using hash (#) character.
Sample Input: 10
Sample Solution:
C Code:
#include<stdio.h>
int main()
{
int size, i, j; // Declare variables for size and loop counters
// Prompt user for the size of the square
printf( "Input the size of the square: \n" );
scanf( "%d", &size );
// Check if the size is within the valid range
if(size < 1 || size > 10) {
printf("Size should be in the range 1 to 10\n"); // Print error message
return 0; // Exit the program
}
// Loop for rows
for(i=0; i<size; i++) {
// Loop for columns
for(j=0; j<size; j++) {
printf(" #"); // Print a space and a #
}
printf("\n"); // Move to the next line after completing a row
}
return 0; // Indicate successful program execution
}
Sample Output:
Input the size of the square: # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #
Pictorial Presentation:
Flowchart:
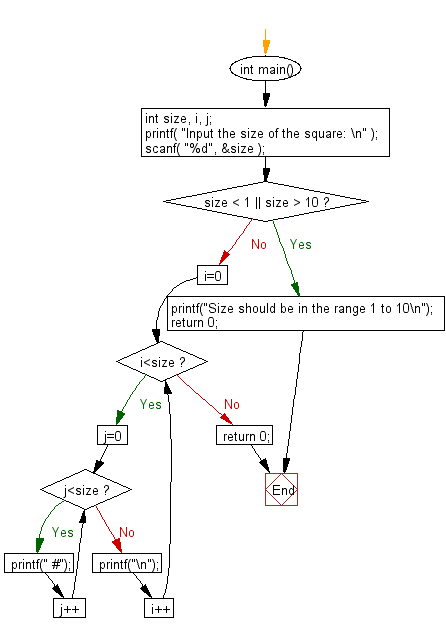
C programming Code Editor:
Previous:Write a C program using looping to produce the following table of values.
Next: Write a C program that reads the side (side sizes between 1 and 10 ) of a square and prints a hollow square using hash (#) character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics